Tips: prepare your data with free JSON validators
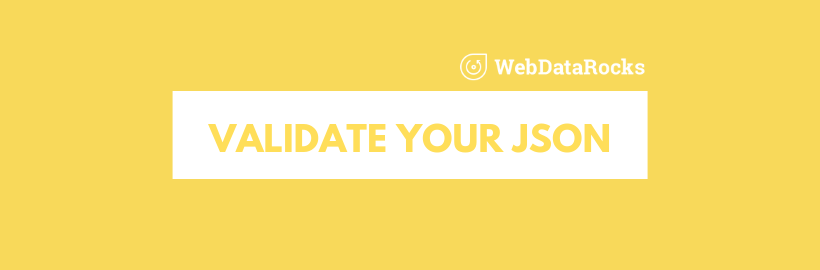
JSON stands for JavaScript Object Notation. It’s a syntax that allows storing information in a self-explanatory and readable manner. Any JSON data can be converted into JavaScript objects. And vice versa: you can convert a JavaScript object into JSON before sending it to a server. This is what makes it so powerful and irreplaceable for fast server-to-browser communication.
Why JSON
It’s favored by developers for its comprehensible structure. A JSON object contains key-value pairs which are called properties. The properties are separated by commas. You can access any property by referring to its name. Each object is surrounded by curly braces.
JSON for reporting
To work with WebDataRocks, you need to pass an array of JSON objects to the reporting tool.
But before loading your JSON data into the pivot table, you should make sure it’s valid.
To prevent any issues with your data, we recommend using JSON validators – special online web services that allow you to load your JSON and check whether it contains any errors. Therefore, we’ve carefully prepared for you an overview of the best JSON validators.
Instead of scrolling hundreds or thousands of lines of code so as find an error, use them. Not only they save a great amount of your time but also format your dataset accurately.
JSONLint
JSONLint is one of the most popular free validators and formatters for JSON data. It’s incredibly simple to use.
To validate your data, follow these steps:
- Paste the JSON data or a link to it into the input field.
- Click “Validate JSON”.
- Read a message – it tells whether there is a problem with your JSON and if so, it specifies the line where the error occurred.
- After correcting the errors, copy the formatted JSON data.
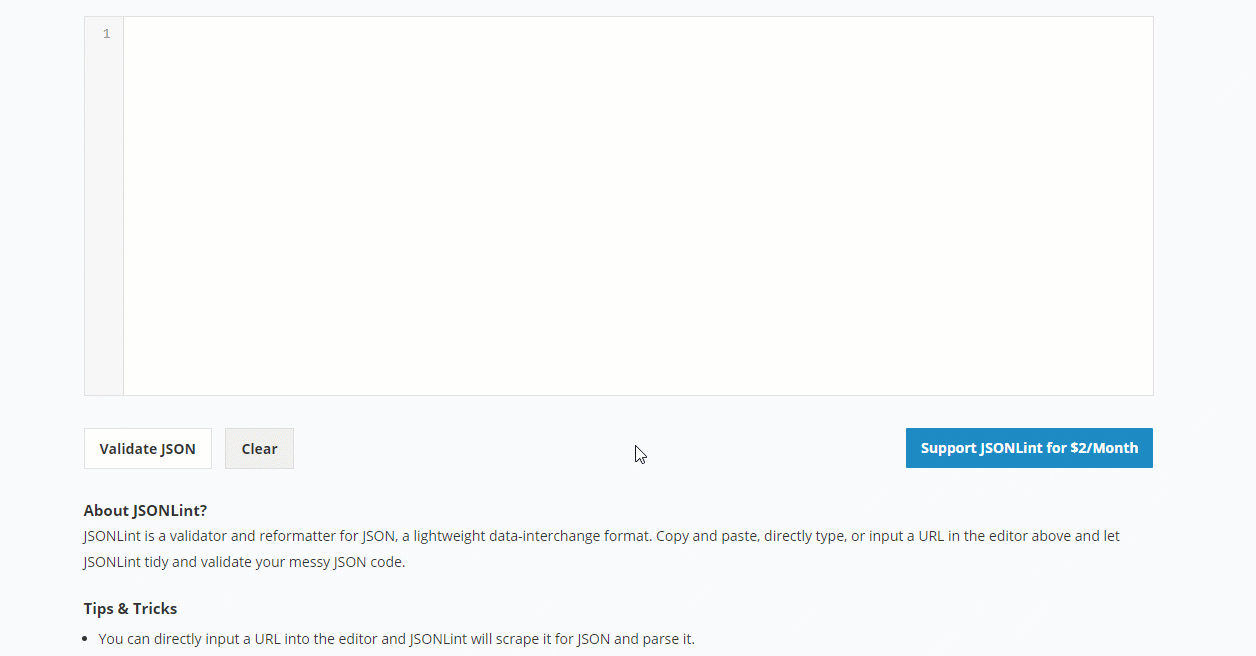
Now your data is ready to be loaded into a pivot grid.
JSON formatter
This free and powerful tool helps you validate, beautify and minify your JSON data. Along with validating functionality, it comes with extra features: you can set indentation levels, print the formatted data, convert it to CSV, YAML or XML formats and download the data into a file. Also, it’s a great option if you have nested JSON objects as it enables you to see the tree view of your data. We tested this validator on browser compatibility – everything works as expected in Chrome, Safari, Edge and Firefox browsers.
How to validate your data:
- Load the data by entering the URL of the file, pasting the data into the input field or browsing the file from your local storage.
- Click “Validate”.
- Choose tabs indentation, format your data and copy the resulting dataset or simply download it.
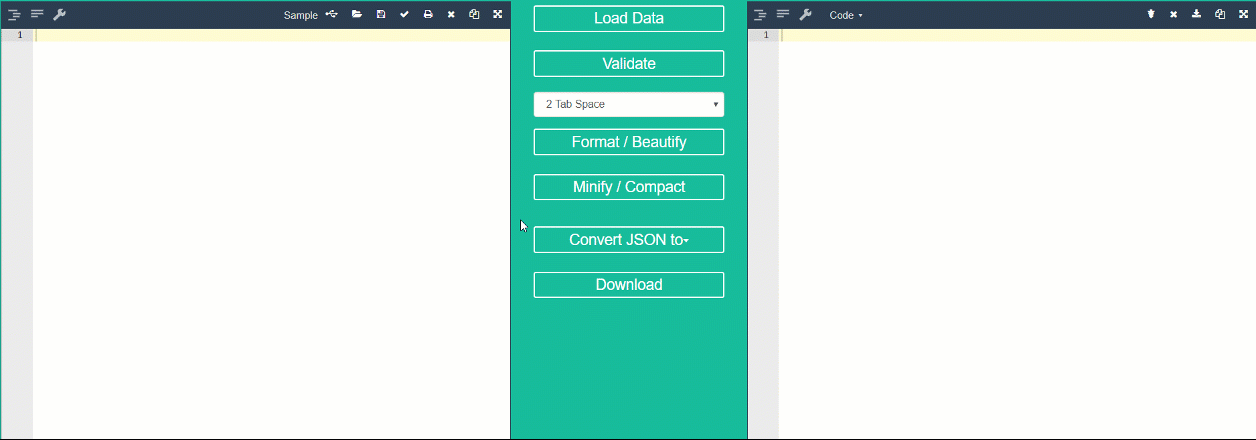
Summary
Preparing your data is the most important step on the way to successful data analysis. We hope these tools will make working with your data a trouble-free process.
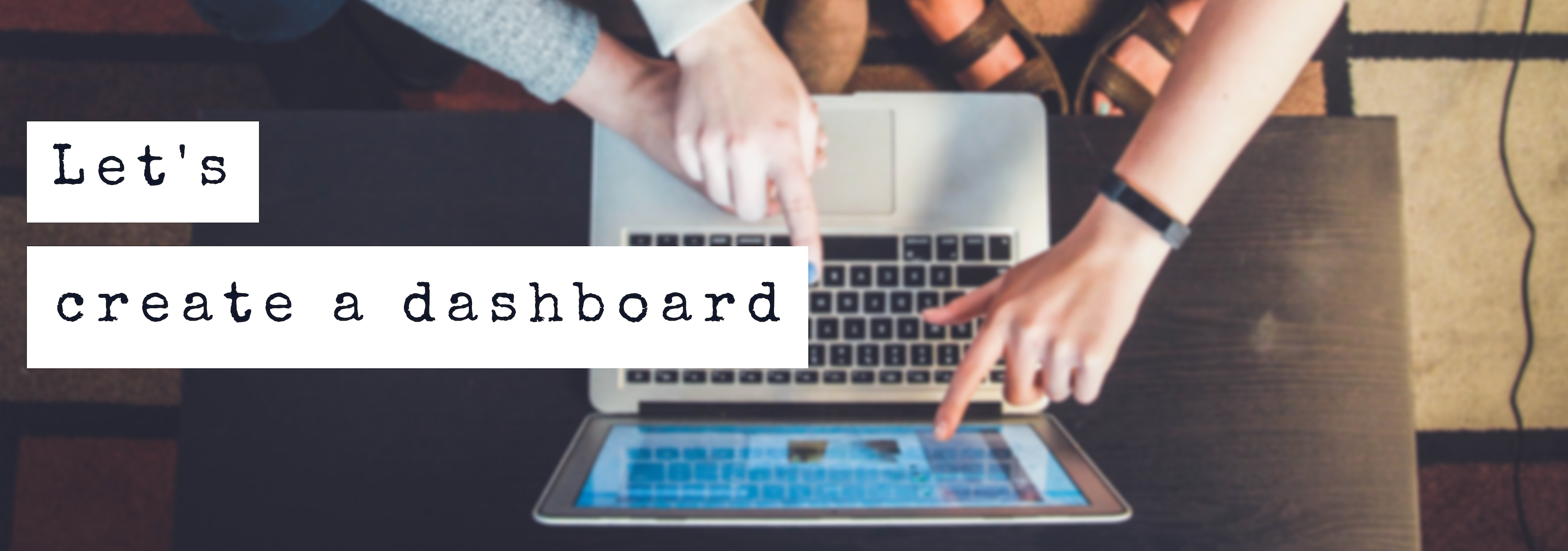
When you use static reports to make up a baseline for your data analysis you should always keep in mind that they show only the static view of your data. To take a look at it in real time, the majority of data analysts opt for dashboards. The reason for that is because they provide more opportunities for exploring the data interactively, meaning you can always go deeper into the details or get the big picture.
Challenges
As a developer, one day you may receive a task to configure a dashboard and embed it into the business application. After you’ve found suitable tools, a lot of heavy-lifting may be involved in the integration process: compatibility with the software stack, customization challenges, overall performance, and, of course, budget limitations.
Also, since dashboards are powered by data which often comes from multiple sources, end-users should be able to connect it to the dashboard, switch between data sources and present the data in a consumable form as a result. The whole process needs to be completely time-saving.
What is the solution?
That’s where free JavaScript libraries come to the rescue.
WebDataRocks is a reporting tool that allows aggregating, filtering and sorting the data.
Google Charts is a data visualization library that provides with a wide range of charts and graphs.
With their help, you can build a low-cost yet effective solution for any analytical project with any front-end technology including Angular, AngularJS, jQuery and React. Both tools are lightweight and extremely customizable.
Today we will focus on creating a fully personalized dashboard for monitoring sales metrics. If you are eager to get hands-on experience, scroll down to the section with useful links and run the demo. It will be enriched with the dynamic report and responsive charts. We truly hope this tutorial will help you get a better understanding of how to combine the analytical features of a pivot table and the expressive power of charts.
Ready?
Let’s start!
Step 1: Define your problem & objectives
What is a data analysis without a purpose? Setting your goals from the very beginning is a milestone on the road to successful analysis. It’s better to have a clear idea in mind of what your end-users need to achieve in the long run.
For an illustrative example, let’s analyze the data of a fictional supply chain to understand its sales performance on a monthly basis as well as customer demographics.
Step 2: Add a reporting tool
A pivot table is an engine of a dashboard as it takes all your raw data and transforms into the summarized form.
Firstly, establish all the dependencies of WebDataRocks and include its styles into the <head>
of your web page:
<link href="https://cdn.webdatarocks.com/latest/webdatarocks.min.css" rel="stylesheet"/>
<script src="https://cdn.webdatarocks.com/latest/webdatarocks.toolbar.min.js"></script>
<script src="https://cdn.webdatarocks.com/latest/webdatarocks.js"></script>
Secondly, create a container which will hold a pivot table:
<div id="wdr-component"></div>
Thirdly, add the component to your web page:
var pivot = new WebDataRocks({ container: "#wdr-component", toolbar: true });
Step 3: Prepare and load your data
Pull the CSV or JSON data from your database, CRM or any other data source.
You have three equivalent options of loading it into the pivot table:
- By specifying a URL to your data file:
"dataSource": { "dataSourceType": "json", "filename": "URL-to-your-data" }
- By connecting to a data source via the UI:
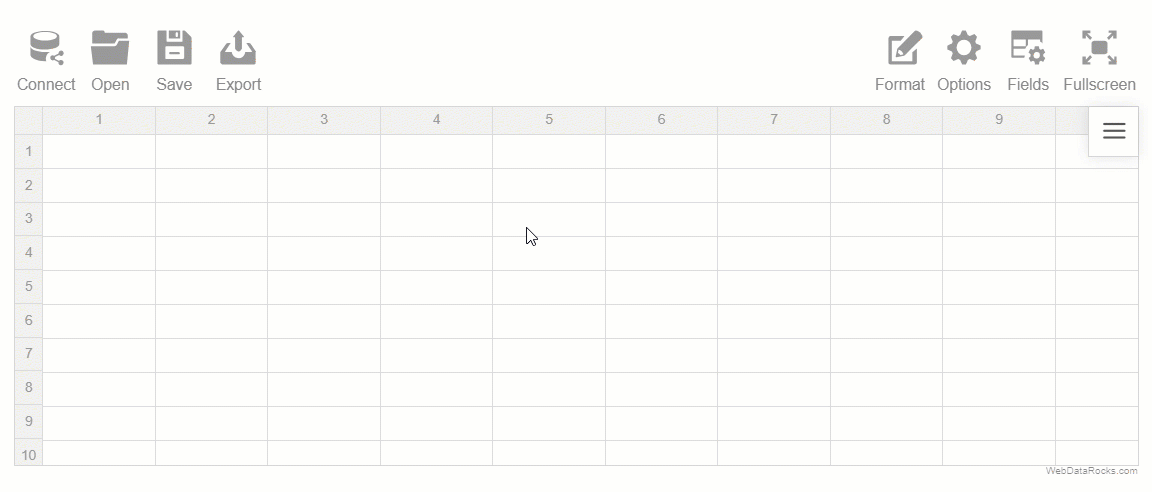
- By defining a function which returns the JSON data.
"dataSource": { "data": getJSONData() }
Step 4: Create a report and aggregate the data
Firstly, set up a slice – it’s the most important part of the report where you specify which hierarchies to place into the rows and columns, apply various filtering and pick aggregation functions for your measures.
To extend the measuring capabilities, calculated values are at your disposal:
- Put the ‘Location’ hierarchy into the rows and ‘Product Group’ into the columns.
- Place the ‘Product’ hierarchy under ‘Product Group’ so as to be able to expand the latter to get more details.
- Create the ‘Sales’ calculated value and put it to the measures. It will help you track the revenue of our organization.
Please refer to the source code at the end of the article to learn how to set a slice.
Step 5: Connect to the charting library
Now that you aggregated data and displayed it on the grid, connect to the Google Charts library by including its Visualization API loader into the <head>
of your web page:
<script src="https://www.gstatic.com/charts/loader.js"></script>
After that, load the package for particular types of charts (either in the <head>
or <body>
section):
google.charts.load('current', {
'packages': ['corechart']
});
Also, you need to add the WebDataRocks connector for Google Charts that handles all the data pre-processing for the specific types of charts:
<script src="https://cdn.webdatarocks.com/latest/webdatarocks.googlecharts.js"></script>
Lastly, add containers for the charts:
<div id="combo-chart-container"></div> <div id="pie-chart-container"></div> <div id="bar-chart-container"></div>
Step 6: Bind the charts to the pivot table
To make the charts show the data from the table, you need to attach an event handler to the pivot table for the reportcomplete
event.
reportcomplete: function() { pivot.off("reportcomplete"); pivotTableReportComplete = true; createComboChart(); createPieChart(); createBarChart(); }
To track the pivot’s and Google Charts readiness, add two flag variables:
var pivotTableReportComplete = false;
var googleChartsLoaded = false;
Also, set the onGoogleChartsLoaded()
function as a callback to run after the “
google.charts.load('current', { 'packages': ['corechart'] }); google.charts.setOnLoadCallback(onGoogleChartsLoaded);
onGoogleChartsLoaded()
is responsible for getting the data from the pivot and starting the functions that instantiate our charts:
function onGoogleChartsLoaded() { googleChartsLoaded = true; if (pivotTableReportComplete) { createComboChart(); createPieChart(); createBarChart(); } } function createComboChart() { if (googleChartsLoaded) { pivot.googlecharts.getData({ type: "column" }, drawComboChart, drawComboChart); } } function drawComboChart(_data) { var data = google.visualization.arrayToDataTable(_data.data); var options = { title: _data.options.title, height: 300, legend: { position: 'top' } }; var chart = new google.visualization.ComboChart(document.getElementById('combo-chart-container')); chart.draw(data, options); }
Similarly, you need to define the functions for a pie and a bar chart.
In the definition of each function, specify the chart types as the input arguments for
As the second and the third arguments, pass the functions that act as a callback and update handlers, meaning they are called once the data is loaded into the pivot table or updated. This way we make the chart react to the tiniest changes in our report.
Also, don’t forget to set the charts options to make them look awesome.
Step 7: Apply extra options
Let’s make our dashboard even more expressive and customize the grid cells based on the values.
With the customizeCell
function, we’ll redefine the style and content of the cells with totals.
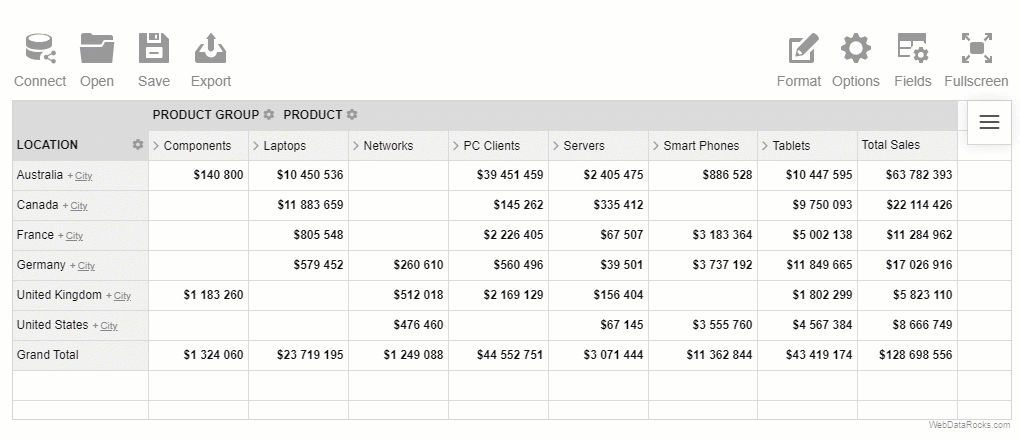
Now we can clearly observe the trends in our sales data, can’t we?
Step 8: Enjoy the result
Summarizing all the steps, today you’ve learned in practice how to build a sales dashboard with minimum code, maximum flexibility, and effectiveness. Now you can share it with your team and help them reveal deeper insights from the data.
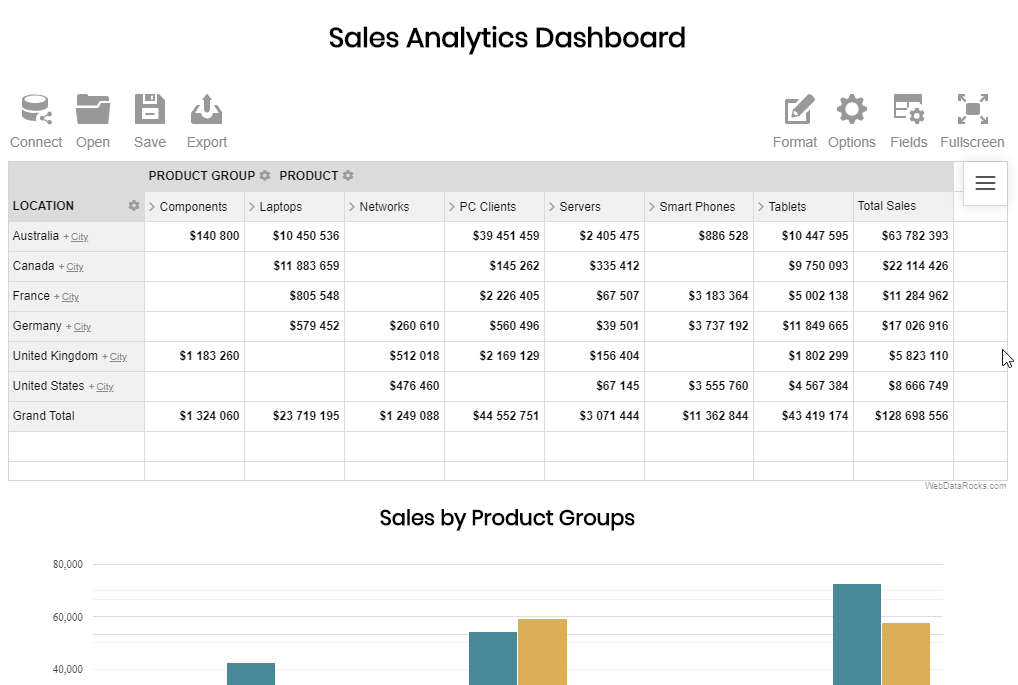
See a complete code in the live demo.
You can always tune the report and get the new view of your data in real-time – simply drag and drop the hierarchies to switch them, expand or collapse their members and drill through the records to see the raw data and reveal more insights. Feel free to add the report filters to focus on the months in which you are interested the most.

- Sales Dashboard Demo
- WebDataRocks Quick Start Guide
- Google Charts Quick Start Guide
- How to integrate WebDataRocks Pivot Table with Google Charts
- JavaScript tutorial on GitConnected for deepening your knowledge in JavaScript
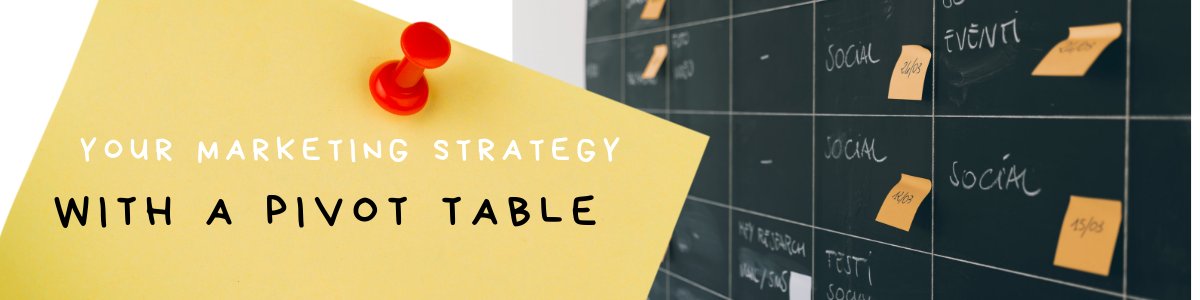
It is well known that digital marketing is an irreplaceable way to connect and communicate online with potential customers of your business with the help of different channels, both free and paid. Its set of tactics comprises diverse tools such as search engine marketing, email marketing, posting content to social media, remarketing, which help stay engaged with prospects and much more. When combined together, they are called integrated marketing methods and help achieve the most sophisticated goals.
According to Gartner’s Digital Marketing Spend report, marketers invest 25% of marketing expense budget in digital marketing methods as they remain the most effective tactics to convert traffic into leads, subscribers, and sales.
To make the investments profitable, the first and foremost rule for a marketer is to plan the campaign thoroughly and analyze results afterward. Only thus can the efforts be rewarding.
Where to get the data?
Data is the heart of any analysis. And marketing analysis is no exception.
There are plenty of ways of storing marketing data and pulling it out – from databases to analytical platforms. Not all of them are suitable for reporting and data analysis, though. By blending the data from various sources, you can collect a versatile dataset to work with.
For you, as a marketer, keeping the analytics in one place is vital. Having finished your campaign and collected enough data, it’s the best time to put your raw data to work. That’s exactly where a pivot table may stand you in good stead.
Pivot Table Magic for Marketing Analysis
Recently we’ve sorted out the structure and purpose of the pivot table as a powerful tool for web reporting. Now it is the best time to find out how it may come in handy for shaping your marketing strategy.
Today we’ll analyze and measure paid marketing campaigns: track their results and evaluate the performance so as to maximize the return on the investment of time and money.
What metrics to consider?
For this, we should take a look at trends in the engagement metrics (such as conversion rates, sessions, bounce rates) and the overall amount of leads, opportunities, and sales over a certain time period (let’s say, six months).
When you think of metrics, naturally you imagine a performance dashboard. That’s why we’ll try a mixed technique that lies in combining reporting features of a pivot table and visualization capabilities of charts.
How to calculate performance measures
- Revenue = Total Sales * Purchase Cost
- Gross Profit = Revenue – Campaign Cost
- ROI (return on investment) = (Gross Profit – Campaign Cost) / Campaign Cost
- Conversion Rate = Total Leads / Total Clicks
- CPL (Cost Per Lead) = Total Marketing Spend / Total New Leads
- CPO (Cost Per Opportunity) = Total Marketing Spend / Total Opportunities
- CPS (Cost Per Sale) = Total Marketing Spend / Total Sales
Methods of calculating these metrics depend greatly on the specifics of the industry you’re working in, that’s why your formulas may differ from ours. The purpose of this article is to show how you can take advantage of pivot table capabilities for effective analysis of various metrics.
Goals of analysis
Before the start, we should know what results we want to achieve in the long run.
Our far-reaching goal is to optimize leads-opportunities and opportunities-sales conversions.
That’s why we’ll pay particular attention to amounts of leads, opportunities, and sales generated by channels and campaigns. Also, we’ll calculate ROI, CPL, CPO, and CPC as these metrics are the foundation of measuring how successful the campaign was. ROI is of special importance – it shows how profitable the marketing investment was.
We’ll show you how to analyze real live data and provide you with the link to the demo at the end of the article.
Reporting
Let’s master the process of creating reports together.
Connect to a data source
Let’s connect to a CSV/JSON data file that has been previously exported from the platform you’re using for marketing.
The fields from our dataset are represented as the hierarchies in the rows, columns, and measures. Particularly, we have the following fields:
- Channel
- Campaign
- Campaign Cost
- Clicks
- CPC
- Date
- Users
- Sessions
- Leads
- Opportunities
- Sales
- Bounce rate
- Purchase Cost
Let’s take a quick glance at the structure of the data in a flat view:
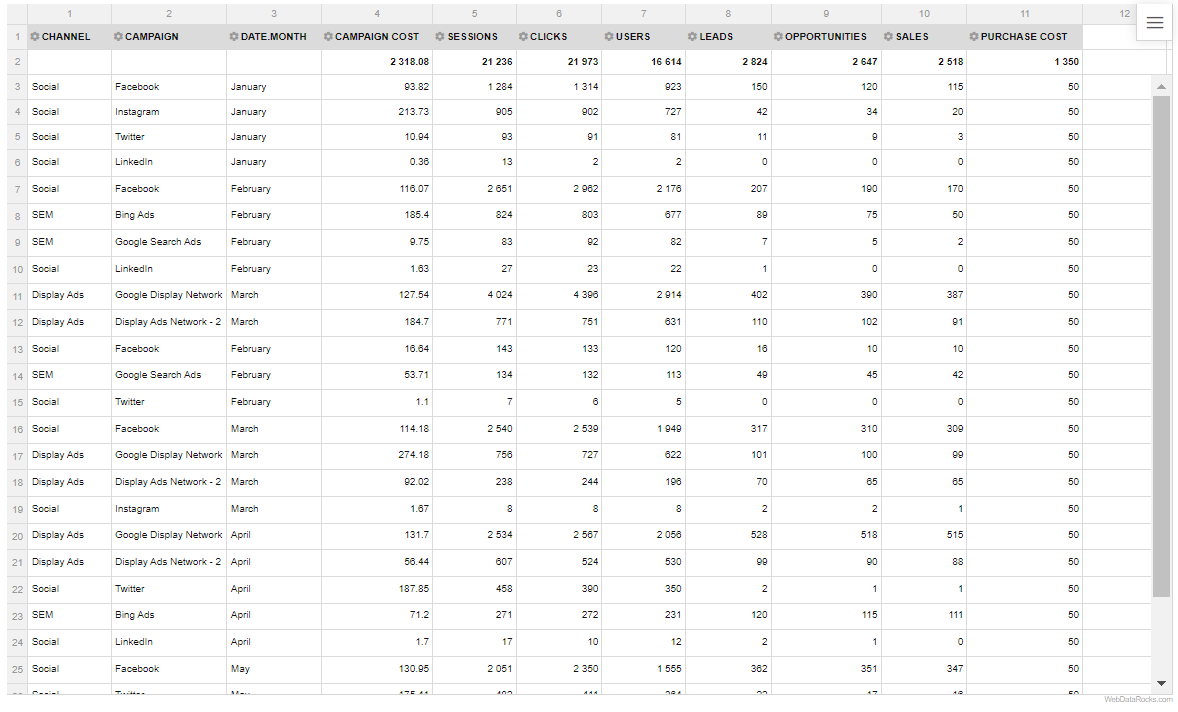
Add custom measures
To get a complete picture of our analysis, let’s add our own metrics by using the calculated values. Simply define the formulas for these measures in the Field List.
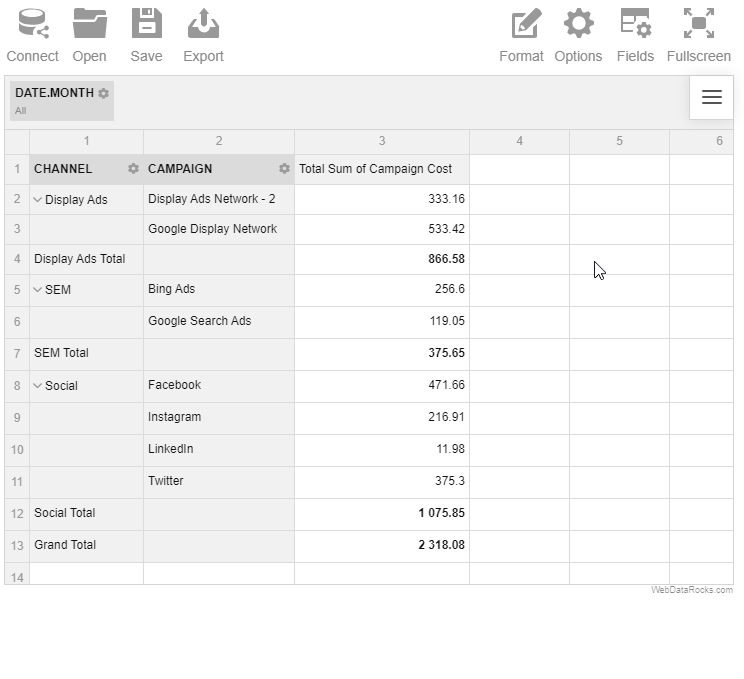
Arrange the hierarchies
Organize the data on the grid and aggregate it via the Field List or by defining a slice in code. Here is the result:
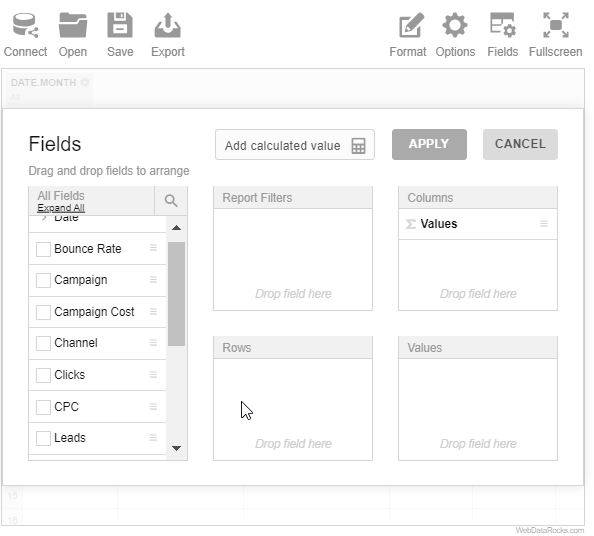
Note that we’ve added the “Month” level of the “Date” hierarchy to the report filters so as to be able to evaluate metrics over specific months.
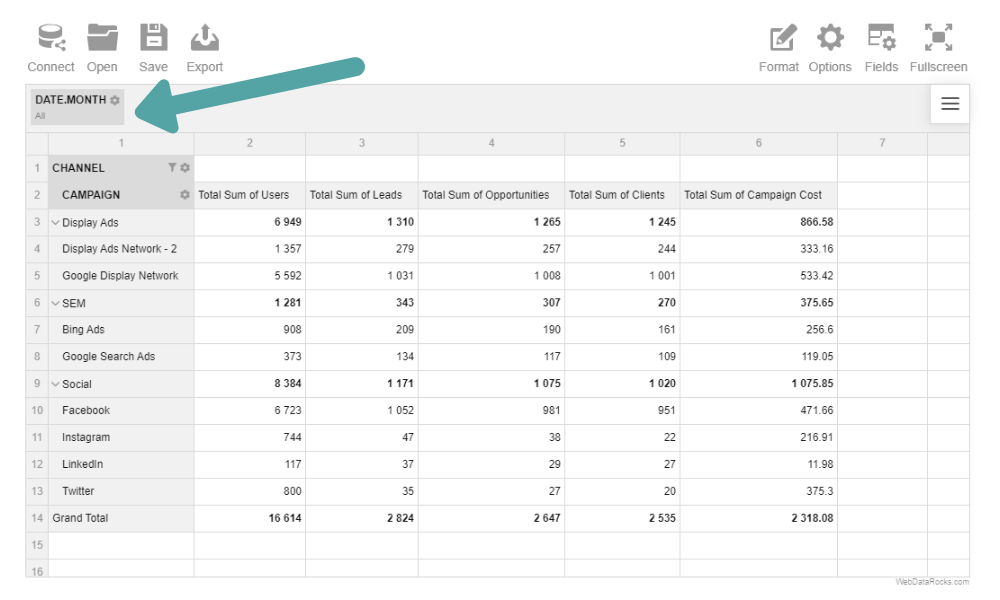
Put the accent on individual cells
To tailor the report, highlight the cells with conditional formatting based on their values:
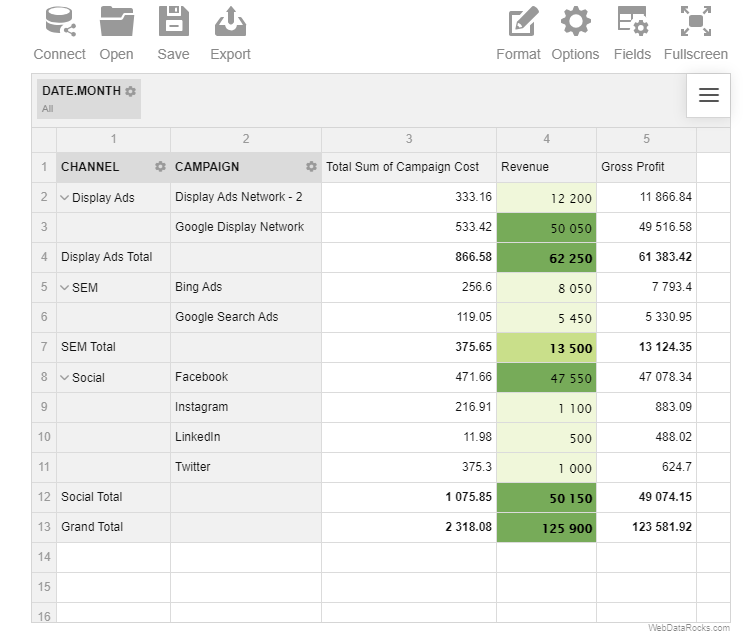
Campaigns Analysis
It’s time to perform a campaigns analysis. Let’s focus on three types of campaigns: Social Media, SEM, and Display Advertising and define which channel is the main driver of traffic to your website.
Social Media Channel
We took the four most popular social media platforms to find out whether social media campaigns have any impact on generating leads.
SEM Channel
Also, we added the results of search engines advertising campaigns to measure their effectiveness:
- Bing Ads
- Google Search Ads
Display Advertising Campaigns
To define how many impressions your display advertising brings and whether your strategy needs improvement, we’ll analyze the flow of traffic from these campaigns:
- Google Display Network
- Display advertisement network – 2
We’ll analyze all this data using a top-down approach: starting from the big picture, we’ll drill down to the deeper levels of detail. In our case, the most logical way is to analyze the metrics of channels and then assess particular campaigns.
Charting
To gain even more insights, you can enhance the report with visual elements such as charts or diagrams that make information easier to grasp.
Let’s add these kinds of graphs to our dashboard:
- Multiple-series line charts for illustrating the trend in the conversion rate over months, comparing clicks, CPL, CPO, and CPS across channels.
- Column charts for comparing the number of leads, opportunities, and sales generated by the campaigns and channels; bounce rates across channels and campaigns and the largest revenue drivers.
And others. All of them are designed to communicate the results of the analysis as effectively as possible. There are no limitations on your choice as we hope you’ll discover your unique way of visualizing the data.
Displaying the results
Now it’s time to estimate what we’ve achieved by following these guidelines.
For example, the next stacked column chart presents how many leads, opportunities, and sales were generated monthly by all the channels:
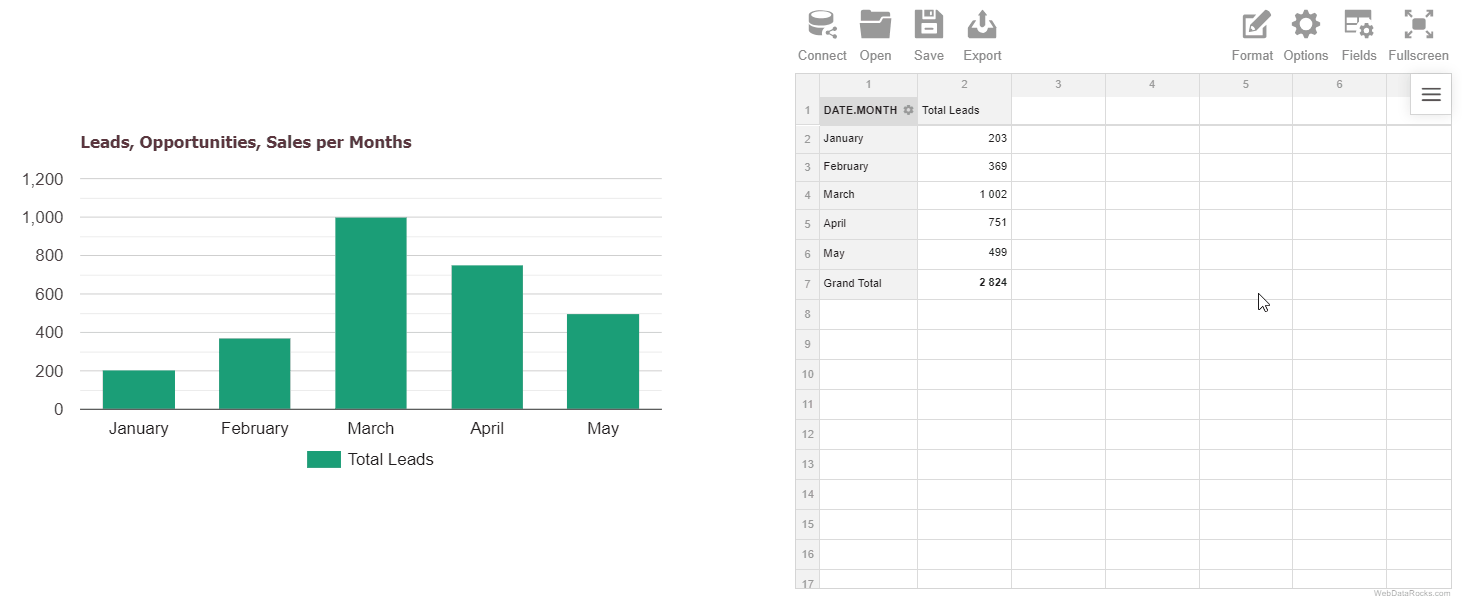
Moreover, we can observe which channels are the drivers of revenue:
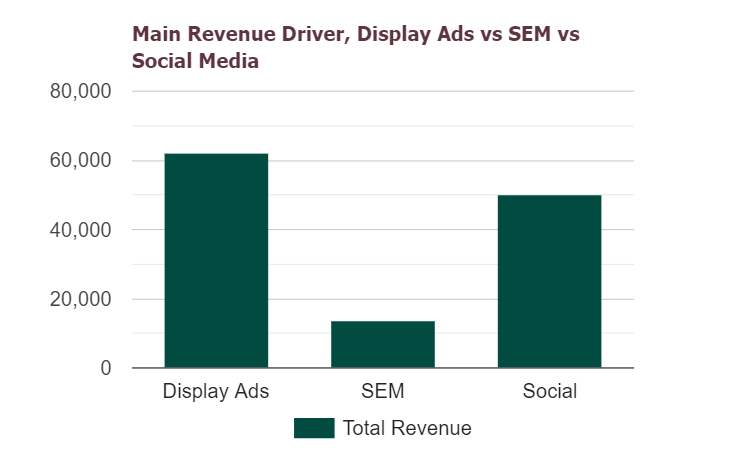
From the chart above it’s clear that the SEM channel brought the least profit and we should analyze Display Ads and Social channels more closely:
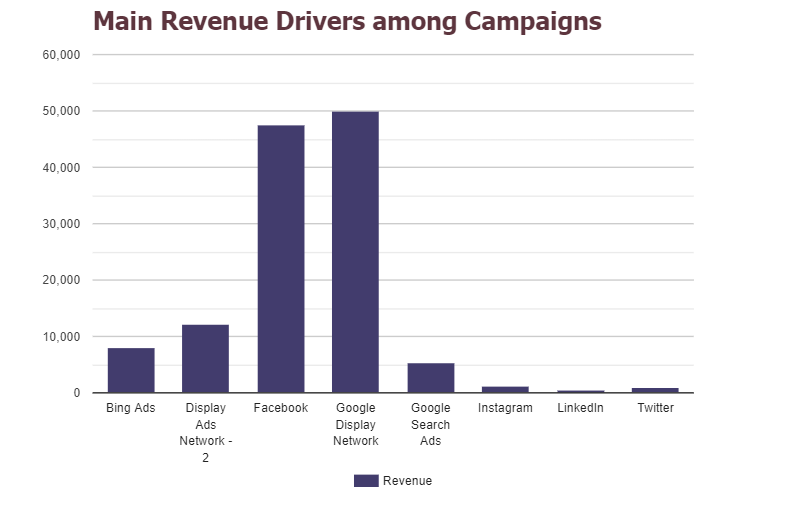
This chart answers the question: “Which campaigns were the most profitable?”
Afterward, let’s dig deeper into the analysis of social media campaigns:
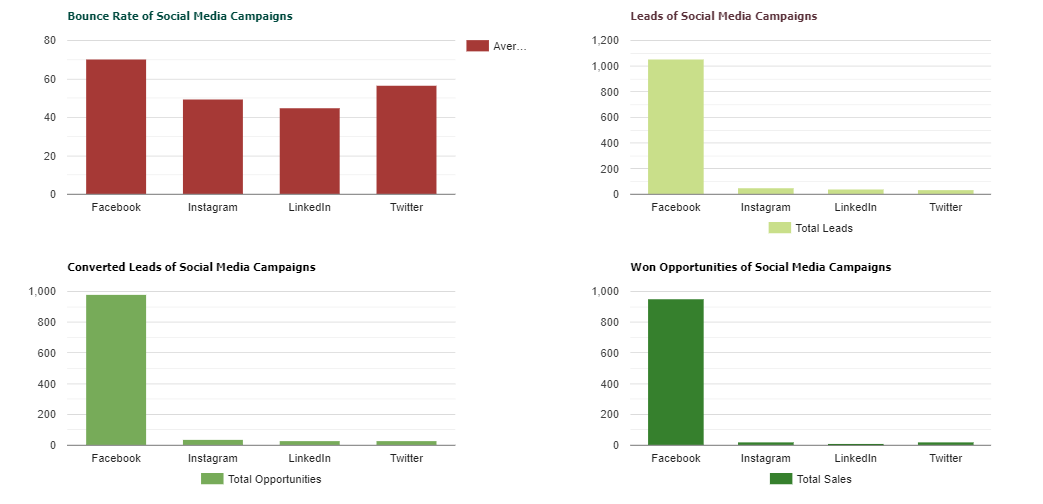
Using these charts, we’ve checked whether the bounce rate was high and how many leads, opportunities and sales were delivered by social media campaigns.
Likewise, you can create interactive dashboards for the rest of the campaigns by using the dashboard templates we’ve carefully prepared for you.
What’s next?
Now that you understand the results of your marketing efforts, feel free to share the report with colleagues or boss.
Bringing it all together
Today you’ve learned how to use a pivot table as a leading tool for doing research on the effectiveness of campaigns. We do hope you are inspired to use it for improving your strategic marketing goals.
Live demo
Experience is the best teacher. We’ve prepared the interactive demos for you – load your own data and try exploring it to reveal more details about your marketing campaigns.
Search the ways to rock the data with us. Stay tuned not to miss new blog posts!
Useful tutorials
- Using conditional formatting
- Integration of WebDataRocks and Google Charts
- Integration of WebDataRocks and Highcharts
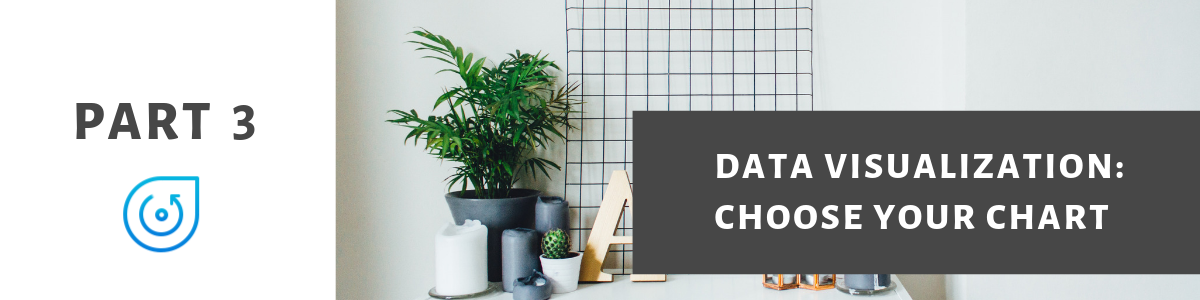
Recently we’ve talked about the basic principles of data visualization and charts for comparing discrete groups of data. Now it’s time to discover charts for one of the most common types of data analysis – a comparison over time.
Today we’ll dive into a process of choosing the comparison charts that display the data over a period of time and help to track trends in changes for one or multiple categories during that period. All of them prove to be extremely helpful in visualizing the performance with respect to time.
The choice of the comparison chart highly depends on how you treat time – as discrete or continuous data. It’s totally up to whether to use bars to focus on individual dates or lines to observe trends over a continuous interval.
So, let’s start!
Line chart
Line charts (also known as trend lines) are best for illustrating trends and volatility in the data. To draw a line chart, plot the data points on a Cartesian plane and connect them with a line. As a rule, time is plotted on the X-axis (as well as any other independent variables) while the values are plotted on the Y-axis.
Purpose
Use it to depict how the data changes over a continuous time period either inside one category or among multiple ones.
Recommendations
- If the sum of values is important, consider using an area chart instead of a line chart.
- Keep it neat: don’t plot more than three-four lines per chart.
Examples and variations
- A single-line chart
Using this chart, you can compare a single metric over time.
Compare the level of sales over the years
- A multi-line chart
Works best for comparing values of multiple categories or groups to one another over time. To distinguish lines by categories and make the chart as readable as possible, plot lines of distinct colors and widths.
- Dual-axis line chart
It is the same as a multi-line chart but with the second Y-axis being added to it. The several usage scenarios are possible:
- For comparing two data series with the same measure which is expressed using different magnitudes (value ranges).
- For comparing two data series which values are expressed with different units of measurement (e.g., in degrees Celsius and Fahrenheit, in degrees and hours, etc).
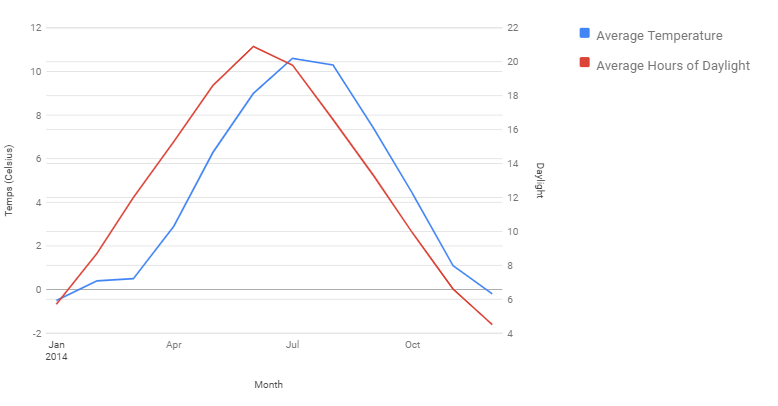
Column chart
Column chart consists of vertical bars the lengths of which are proportional to the values. It’s suitable not only for comparing the data between various categories but also for showing trends for the same or multiple measures over discrete time intervals.
Recommendation
If you have long data labels or/and a large number of data-series, it’s better to use a bar chart instead as it uses space more rationally.
Example
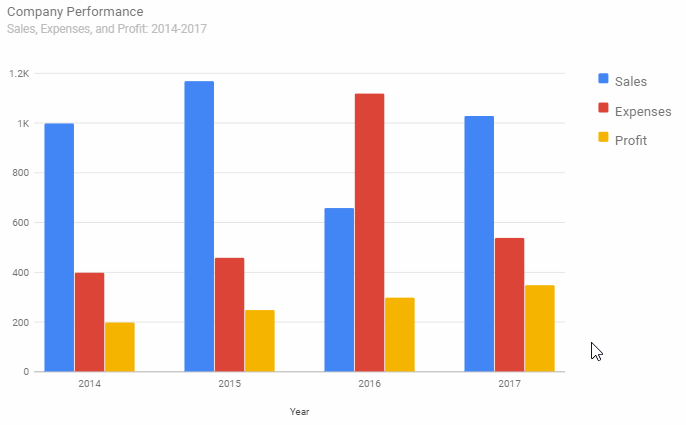
Comparing the company’s sales, expenses, and profit over the years
Step chart
A step chart is also known as a step graph. Its main difference from a line chart lies in a method of connecting the points. Instead of connecting the points using the shortest distance between them, it plots vertical and horizontal lines to connect the data points on a plane. Vertical lines can be interpreted as abrupt changes in time-series data.
Purpose
Use it to track the discrete changes in the data that happen at specific moments of time.
Recommendations
- Use it with a timestamp data, or with one that has missing values for some dates.
- To communicate the magnitude of change, fill an area under the line with color.
Example
Compare stock prices by days
Area chart
This chart is graphed on the X- and Y-axis, with values being connected using line segments. The area between line segments and axes is filled with color.
Purpose
Use it to accentuate on how the magnitude of the cumulative value evolves over time.
Recommendations
- Assign contrast colors to different data series to help your audience compare values at glance.
- When communicating trends of multiple categories, avoid overlapping plots – this representation may be misleading for your audience. Prefer using stacked area charts instead.
- If the differences between the successive values are tiny, give preference to a line chart.
Examples and variations
- A single-series area chart
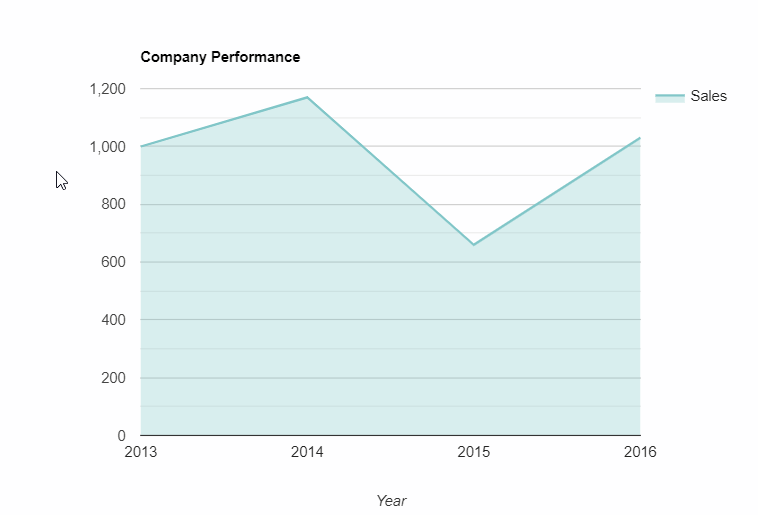
- A multi-series area chart
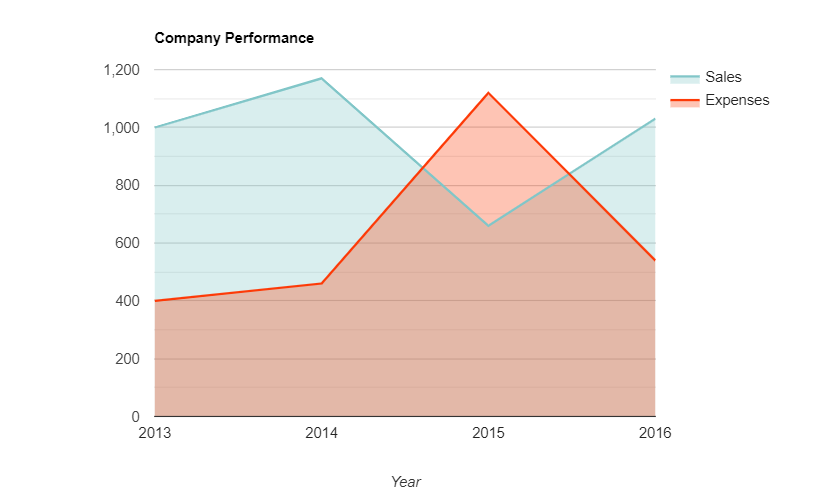
- A stacked area chart
The elements for data series are stacked at each value and are rescaled to add up to 100%. This extension of the area chart is more readable, isn’t it?
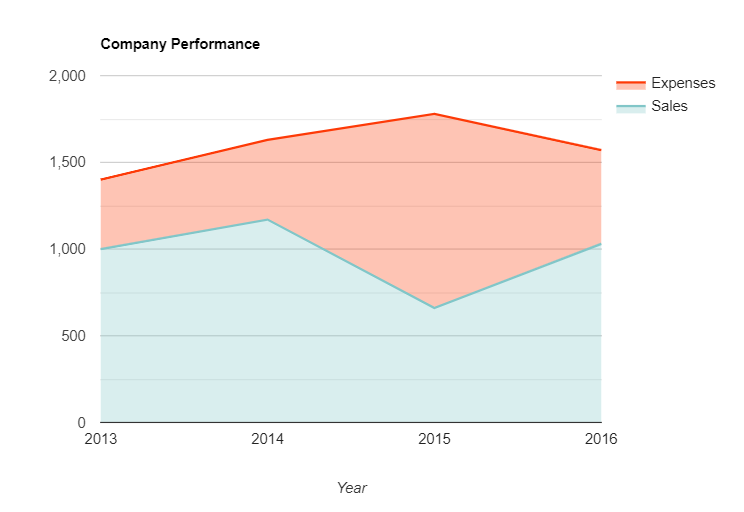
Summary
At WebDataRocks, not only we enhance your reporting experience but share knowledge about the best modern visualization techniques.
Hopefully, this third step of our data visualization journey helps to deepen your knowledge of the charts. We are sure that all of them deserve to take a worthy place in your presentation.
What’s next?
To keep up with all the contributions to our project, check out all the blog posts on the data visualization topic:
- Power of Data Visualization and Charts
- Best Charts to Show Discrete Data
- How to Choose Charts to Show Data Composition
- Best Charts to Show Correlation
- Best Charts to Show Data Distribution
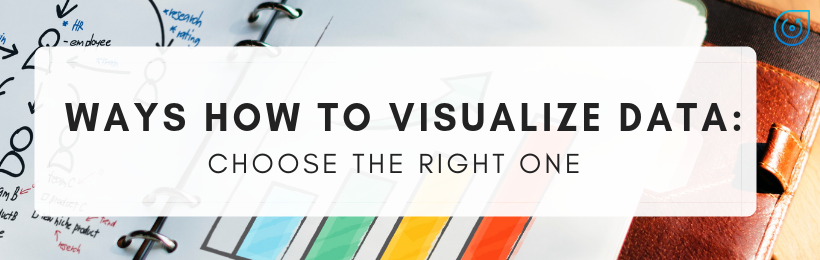
Numerous researches in the field of cognitive neuroscience show that your brain loves a visual content more than plain text.
(more…)Being a web component it’s important to be easily fittable to any web project.
WebDataRocks is as customizable as it’s required by the application where it is embedded.
When you are creating your website or application, the design of the user interface comes as an important part of the developing process. (more…)
We understand your JSON data may contain different types of fields: numbers, dates, strings, time, days of the week and many others!
Setting proper data types at the very beginning is important for correct aggregation in the pivot table and successful data analysis.
Let WebDataRocks take care of handling data types in your JSON dataset. (more…)
There are no insignificant details when it comes to the design of your application. That’s why our team pays attention to every little detail and provides an opportunity to define how your reports will look and feel.
(more…) class=”alignnone size-full wp-image-990″ />
As we appreciate the importance of personalization for you, we cannot allow the component to stand out from the general color scheme of your application. (more…)
Bring the brightness to your data
Do you want to make your web report look more interactive and appealing?
To emphasize on the visual representation of your data you are able to use any of the most popular charting libraries – Google Charts, Highcharts, FusionCharts or any third-party charting library you prefer.
(more…)