Integration with any charting library (React)
This tutorial explains how to connect WebDataRocks to a third-party charting library, such as Chart.js, ApexCharts, ECharts, or D3.js.
WebDataRocks can be integrated with charting libraries using the getData() API call, which returns data displayed on the grid. Then, you need to preprocess the data to a format required by your charting library and pass it to the chart.
In this tutorial, we will integrate with Chart.js, but the steps are similar for other libraries.
Note that if you are integrating with amCharts, Highcharts, FusionCharts, or Google Charts, use our ready-to-use connectors that will automatically process the data. Check out the list of available tutorials.
Step 1. Add WebDataRocks to your project
Step 1.1. Complete the integration guide. Your code of the component with WebDatarocks should look similar to the following:
import * as WebDataRocksReact from "@webdatarocks/react-webdatarocks";
function App() {
return (
<div>
<WebDataRocksReact.Pivot
toolbar={true}
/>
</div>
);
}
export default App;
Step 1.2. Create a report for WebDataRocks — connect to the data source and define which fields should be displayed in rows, columns, and measures:
function App() {
const report = {
dataSource: {
filename: "https://cdn.webdatarocks.com/data/data.csv",
},
slice: {
rows: [
{
uniqueName: "Country",
},
],
columns: [
{
uniqueName: "Measures",
},
],
measures: [
{
uniqueName: "Price",
aggregation: "sum",
},
],
},
};
return (
<div>
<WebDataRocksReact.Pivot
toolbar={true}
report={report}
/>
</div>
);
}
The fields you’ve specified in the report will be shown on the chart.
Step 2. Get a reference to the WebDataRocks instance
Some of WebDataRocks methods and events are needed to create a chart. Using a reference to the WebDataRocks instance, we can access WebDataRocks API.
Get the reference with the useRef hook:
React + ES6
// ...
import { useRef } from "react";
function App() {
const pivotRef = useRef(null);
// ...
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
/>
</div>
);
}
export default App;
React + TypeScript
// ...
import { RefObject, useRef} from "react";
function App() {
const pivotRef: RefObject<WebDataRocksReact.Pivot | null> = useRef<WebDataRocksReact.Pivot>(null);
// ...
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
/>
</div>
);
}
export default App;
Now it’s possible to interact with the component through pivotRef.current.webdatarocks
.
Step 3. Add a charting library
Note that this tutorial integrates WebDataRocks with Chart.js. The following steps may vary depending on your charting library.
Step 3.1. Install the npm package with your charting library. For example:
npm i react-chartjs-2 chart.js
Step 3.2. Import the charting library into your component:
import { PolarArea } from "react-chartjs-2";
import {
Chart as ChartJS,
RadialLinearScale,
ArcElement,
Tooltip,
Legend,
} from "chart.js";
ChartJS.register(RadialLinearScale, ArcElement, Tooltip, Legend);
Step 3.3. Add a container for your chart where your React element is returned:
<PolarArea/>
Step 3.4. Create a state variable to store chart data (e.g., chartData
):
React + ES6
import { useRef, useState } from "react";
function App() {
// ...
const [chartData, setChartData] = useState({
labels: [],
datasets: [
{
data: [],
},
],
});
// ...
}
We will pass the data from the component to chartData
in step 4.3.
React + TypeScript
import { RefObject, useRef, useState } from "react";
function App() {
// ...
const [chartData, setChartData] = useState({
labels: [],
datasets: [
{
data: [],
backgroundColor: [
"rgba(255, 99, 132, 0.5)",
"rgba(54, 162, 235, 0.5)",
"rgba(255, 206, 86, 0.5)",
"rgba(75, 192, 192, 0.5)",
"rgba(153, 102, 255, 0.5)",
"rgba(255, 159, 64, 0.5)",],
},
],
});
// ...
}
We will pass the data from the component to chartData
in step 4.3.
Step 3.5. Pass the chart data to the chart container:
<PolarArea data={chartData} />
Step 4. Integrate WebDataRocks with the charting library
In this step, we will use the getData() API call. This method was added especially for integration with third-part charting libraries; using it, you can request data from WebDataRocks and pass it to a charting library.
Step 4.1. If we call the getData() method before WebDataRocks is fully loaded, it will return an empty result. To know when WebDataRocks is ready to provide data for the chart, handle the reportcomplete event:
React + ES6
const onReportComplete = () => {
// Unsubscribing from reportcomplete
// We need it only to track the initialization of WebDataRocks
pivotRef.current.webdatarocks.off("reportComplete");
createChart();
};
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
reportcomplete={onReportComplete}
/>
<PolarArea data={chartData} />
</div>
);
React + TypeScript
const onReportComplete = () => {
// Unsubscribing from reportcomplete
// We need it only to track the initialization of WebDataRocks
pivotRef.current?.webdatarocks.off("reportComplete");
createChart();
};
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
reportcomplete={onReportComplete}
/>
<PolarArea data={chartData} />
</div>
);
Step 4.2. Implement the createChart()
function using the getData() API call:
React + ES6
const createChart = () => {
if (pivotRef.current) {
pivotRef.current.webdatarocks.getData(
{},
// Function called when data for the chart is ready
drawChart,
// Function called on report changes (filtering, sorting, etc.)
drawChart
);
};
};
React + TypeScript
const createChart = () => {
if (pivotRef.current) {
pivotRef.current?.webdatarocks.getData(
{},
// Function called when data for the chart is ready
drawChart,
// Function called on report changes (filtering, sorting, etc.)
drawChart
);
};
};
Step 4.3. Implement the drawChart()
function specified in the previous step. This function will initialize the chart, set all the configurations specific to this chart type, and fill it with the data provided by WebDataRocks:
React + ES6
const drawChart = (rawData) => {
const { labels, values } = prepareData(rawData); // This function will be implemented in step 5
// Configuring the chart and filling it with data
setChartData({
labels: labels,
datasets: [
{
data: values,
backgroundColor: [
"rgba(255, 99, 132, 0.5)",
"rgba(54, 162, 235, 0.5)",
"rgba(255, 206, 86, 0.5)",
"rgba(75, 192, 192, 0.5)",
"rgba(153, 102, 255, 0.5)",
"rgba(255, 159, 64, 0.5)",
],
},
],
});
};
React + TypeScript
const drawChart = (rawData: any) => {
const { labels, values } = prepareData(rawData); // This function will be implemented in step 5
// Configuring the chart and filling it with data
setChartData({
labels: labels,
datasets: [
{
data: values,
backgroundColor: [
"rgba(255, 99, 132, 0.5)",
"rgba(54, 162, 235, 0.5)",
"rgba(255, 206, 86, 0.5)",
"rgba(75, 192, 192, 0.5)",
"rgba(153, 102, 255, 0.5)",
"rgba(255, 159, 64, 0.5)",
],
},
],
});
};
Step 5. Prepare the data
getData() API call returns an object (e.g., rawData
) that contains the data from the grid and its metadata. The metadata includes a number of fields in rows and columns in the slice, an array of format objects, etc. Read more about the getData() response.
Here is an example of the rawData.data
array returned by getData()
. This data is based on the slice that was defined in the step 1.2:
data: [
{ v0: 6221870 }, // Grand total
{ r0: "Australia", v0: 1372281 },
{ r0: "France", v0: 1117794 },
{ r0: "Germany", v0: 1070453 },
{ r0: "Canada", v0: 1034112 },
{ r0: "United States", v0: 847331 },
{ r0: "United Kingdom", v0: 779899 }
]
This raw data now must be transformed to match the data format required by your charting library, in our case — Chart.js. Let’s create a function that will preprocess the data, for example, prepareData()
.
We are passing data to the data.labels
and data.datasets
properties, where data.labels
contains field members and data.datasets
contains values. Read more in the Chart.js documentation.
The prepareData()
function for Chart.js will look similar to the following:
React + ES6
const prepareData = (rawData) => {
const labels = rawData.data.filter((rec) => rec.r0 && rec.v0).map((rec) => rec.r0);
const values = rawData.data.filter((rec) => rec.r0 && rec.v0).map((rec) => rec.v0);
return { labels, values };
};
React + TypeScript
const prepareData = (rawData: any) => {
const labels = rawData.data.filter((rec: any) => rec.r0 && rec.v0).map((rec: any) => rec.r0);
const values = rawData.data.filter((rec: any) => rec.r0 && rec.v0).map((rec: any) => rec.v0);
return { labels, values };
};
prepareData()
must return an object with the data that can be used by your charting library. The example shows the returned object for Chart.js:
{
labels: ["Australia", "France", "Germany", "Canada", "United States", "United Kingdom"],
values: [1372281, 1117794, 1070453, 1034112, 847331, 779899]
}
Step 6. Run the project
Run your project with the following command:
npm run dev
Open http://localhost:5173/
in the browser to see how the pivot table looks in combination with Chart.js.
Try filtering the data, changing measures, and adjusting aggregation functions — the chart will be updated at once.
Check out the full code
After completing this tutorial, the full code of the integration should look as follows:
React + ES6
import { useRef, useState } from "react";
import * as WebDataRocksReact from "@webdatarocks/react-webdatarocks";
import { PolarArea } from "react-chartjs-2";
import {
Chart as ChartJS,
RadialLinearScale,
ArcElement,
Tooltip,
Legend,
} from "chart.js";
ChartJS.register(RadialLinearScale, ArcElement, Tooltip, Legend);
function App() {
const pivotRef = useRef(null);
const [chartData, setChartData] = useState({
labels: [],
datasets: [
{
data: [],
},
],
});
const report = {
dataSource: {
filename: "https://cdn.webdatarocks.com/data/data.csv",
},
slice: {
rows: [
{
uniqueName: "Country",
},
],
columns: [
{
uniqueName: "Measures",
},
],
measures: [
{
uniqueName: "Price",
aggregation: "sum",
},
],
},
};
const onReportComplete = () => {
pivotRef.current.webdatarocks.off("reportcomplete");
createChart();
};
const createChart = () => {
if (pivotRef.current) {
pivotRef.current.webdatarocks.getData(
{},
drawChart,
drawChart
);
};
};
const drawChart = (rawData) => {
const { labels, values } = prepareData(rawData);
setChartData({
labels: labels,
datasets: [
{
data: values,
backgroundColor: [
"rgba(255, 99, 132, 0.5)",
"rgba(54, 162, 235, 0.5)",
"rgba(255, 206, 86, 0.5)",
"rgba(75, 192, 192, 0.5)",
"rgba(153, 102, 255, 0.5)",
"rgba(255, 159, 64, 0.5)",
],
},
],
});
};
const prepareData = (rawData) => {
const labels = rawData.data.filter((rec) => rec.r0 && rec.v0).map((rec) => rec.r0);
const values = rawData.data.filter((rec) => rec.r0 && rec.v0).map((rec) => rec.v0);
return { labels, values };
};
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
reportcomplete={onReportComplete}
/>
<PolarArea data={chartData} />
</div>
);
}
export default App;
React + TypeScript
import { RefObject, useRef, useState } from "react";
import * as WebDataRocksReact from "@webdatarocks/react-webdatarocks";
import { PolarArea } from "react-chartjs-2";
import {
Chart as ChartJS,
RadialLinearScale,
ArcElement,
Tooltip,
Legend,
} from "chart.js";
ChartJS.register(RadialLinearScale, ArcElement, Tooltip, Legend);
function App() {
const pivotRef: RefObject<WebDataRocksReact.Pivot | null> = useRef<WebDataRocksReact.Pivot>(null);
const [chartData, setChartData] = useState({
labels: [],
datasets: [
{
data: [],
backgroundColor: [
"rgba(255, 99, 132, 0.5)",
"rgba(54, 162, 235, 0.5)",
"rgba(255, 206, 86, 0.5)",
"rgba(75, 192, 192, 0.5)",
"rgba(153, 102, 255, 0.5)",
"rgba(255, 159, 64, 0.5)",
],
},
],
});
const report = {
dataSource: {
filename: "https://cdn.webdatarocks.com/data/data.csv",
},
slice: {
rows: [
{
uniqueName: "Country",
},
],
columns: [
{
uniqueName: "Measures",
},
],
measures: [
{
uniqueName: "Price",
aggregation: "sum",
},
],
},
};
const onReportComplete = () => {
pivotRef.current?.webdatarocks.off("reportcomplete");
createChart();
};
const createChart = () => {
if (pivotRef.current) {
pivotRef.current?.webdatarocks.getData(
{},
drawChart,
drawChart
);
};
};
const drawChart = (rawData: any) => {
const { labels, values } = prepareData(rawData);
setChartData({
labels: labels,
datasets: [
{
data: values,
backgroundColor: [
"rgba(255, 99, 132, 0.5)",
"rgba(54, 162, 235, 0.5)",
"rgba(255, 206, 86, 0.5)",
"rgba(75, 192, 192, 0.5)",
"rgba(153, 102, 255, 0.5)",
"rgba(255, 159, 64, 0.5)",
],
},
],
});
};
const prepareData = (rawData: any) => {
const labels = rawData.data.filter((rec: any) => rec.r0 && rec.v0).map((rec: any) => rec.r0);
const values = rawData.data.filter((rec: any) => rec.r0 && rec.v0).map((rec: any) => rec.v0);
return { labels, values };
};
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
reportcomplete={onReportComplete}
/>
<PolarArea data={chartData} />
</div>
);
}
export default App;
See also
This tutorial explains how to connect WebDataRocks to a third-party charting library, such as Chart.js, ApexCharts, ECharts, or D3.js.
WebDataRocks can be integrated with charting libraries using the getData() API call, which returns data displayed on the grid. Then, you need to preprocess the data to a format required by your charting library and pass it to the chart.
In this tutorial, we will integrate with Chart.js, but the steps are similar for other libraries. Our integration is based on the Chart.js Getting started guide.
Note that if you are integrating with amCharts, Highcharts, FusionCharts, or Google Charts, use our ready-to-use connectors that will automatically process the data. Check out the list of available tutorials.
Step 1. Add WebDataRocks to your project
Step 1.1. Complete the integration guide. Your code of the web page with WebDatarocks should look similar to the following:
<html>
<head>
<script src="https://cdn.webdatarocks.com/latest/webdatarocks.js"></script>
<script src="https://cdn.webdatarocks.com/latest/webdatarocks.toolbar.min.js"></script>
<link href="https://cdn.webdatarocks.com/latest/webdatarocks.min.css" rel="stylesheet"/>
</head>
<body>
<div id="pivotContainer"></div>
<script>
const pivot = new WebDataRocks({
container: "#pivotContainer",
toolbar: true,
});
</script>
</body>
</html>
Step 1.2. Create a report for WebDataRocks — connect to the data source and define which fields should be displayed in rows, columns, and measures:
const pivot = new WebDataRocks({
container: "#pivotContainer",
toolbar: true,
report: {
dataSource: {
filename: "https://cdn.webdatarocks.com/data/data.csv",
},
slice: {
rows: [
{
uniqueName: "Country",
},
],
columns: [
{
uniqueName: "Measures",
},
],
measures: [
{
uniqueName: "Price",
aggregation: "sum",
},
],
},
},
});
The fields you’ve specified in the report will be shown on the chart.
Step 2. Add a charting library
Step 2.1. Include the scripts for the charting library into your web page:
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
Step 2.2. Add a container to your web page where the chart should be rendered:
<div style="width: 700px;">
<canvas id="chartContainer"></canvas>
</div>
Step 3. Integrate WebDataRocks with the charting library
In this step, we will use the getData() API call. This method was added especially for integration with third-part charting libraries; using it, you can request data from WebDataRocks and pass it to a charting library.
Step 3.1. If we call the getData() method before WebDataRocks is fully loaded, it will return an empty result. To know when WebDataRocks is ready to provide data for the chart, handle the reportcomplete event:
const pivot = new WebDataRocks({
container: "#pivotContainer",
toolbar: true,
report: {
// ...
},
reportcomplete: function() {
pivot.off("reportcomplete");
createChart();
}
});
Now the chart will be created only when the data is loaded and the report is ready.
Step 3.2. Declare a variable for the chart:
let chart;
Step 3.3. Implement the createChart()
function using the getData() API call:
function createChart() {
pivot.getData(
{},
// Function called when data for the chart is ready
drawChart,
// Function called on report changes (filtering, sorting, etc.)
updateChart
);
};
Step 3.4. Implement the drawChart()
function specified in the previous step. This function will initialize the chart, set all the configurations specific to this chart type, and fill it with the data provided by WebDataRocks:
function drawChart(rawData) {
let chartData = prepareData(rawData); // This function will be implemented in step 4
// Configuring the chart
const config = {
type: "polarArea",
data: {
labels: chartData.labels,
datasets: [{
data: chartData.values,
}]
},
};
// Creating a new chart instance
chart = new Chart(
document.getElementById("chartContainer"),
config
);
};
Step 3.5. Implement the updateChart()
function specified in step 3.3. This function will be called on the report changes (e.g., filtering, sorting, etc.):
function updateChart(rawData) {
chart.destroy();
drawChart(rawData);
};
Step 4. Preprocess the data for the chart
getData() API call returns an object (e.g., rawData
) that contains the data from the grid and its metadata. The metadata includes a number of fields in rows and columns in the slice, an array of format objects, etc. Read more about the getData() response.
Here is an example of the rawData.data
array returned by getData()
. This data is based on the slice that was defined in the step 1.2:
data: [
{ v0: 6221870 }, // Grand total
{ r0: "Australia", v0: 1372281 },
{ r0: "France", v0: 1117794 },
{ r0: "Germany", v0: 1070453 },
{ r0: "Canada", v0: 1034112 },
{ r0: "United States", v0: 847331 },
{ r0: "United Kingdom", v0: 779899 }
]
This raw data now must be transformed to match the data format required by your charting library, in our case — Chart.js. Let’s create a function that will preprocess the data, for example, prepareData()
.
We are passing data to the data.labels
and data.datasets
properties, where data.labels
contains field members and data.datasets
contains values. Read more in the Chart.js documentation.
The prepareData()
function for Chart.js will look similar to the following:
function prepareData(rawData) {
let labels = rawData.data.filter(rec => rec.r0 && rec.v0).map(rec => rec.r0);
let values = rawData.data.filter(rec => rec.r0 && rec.v0).map(rec => rec.v0);
return {
labels: labels,
values: values
};
};
prepareData()
must return an object with the data that can be used by your charting library. The example shows the returned object for Chart.js:
{
labels: ["Australia", "France", "Germany", "Canada", "United States", "United Kingdom"],
values: [1372281, 1117794, 1070453, 1034112, 847331, 779899]
}
Step 5. See the result
Open your web page in the browser to see how the pivot table looks in combination with your charting library.
Try filtering the data, changing measures, and adjusting aggregation functions — the chart will be updated at once.
Check out the full code
After completing this tutorial, the full code of the web page should look as follows:
<html>
<head>
<script src="https://cdn.webdatarocks.com/latest/webdatarocks.js"></script>
<script src="https://cdn.webdatarocks.com/latest/webdatarocks.toolbar.min.js"></script>
<link href="https://cdn.webdatarocks.com/latest/webdatarocks.min.css" rel="stylesheet"/>
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
</head>
<body>
<div id="pivotContainer"></div>
<div style="width: 700px;">
<canvas id="chartContainer"></canvas>
</div>
<script>
const pivot = new WebDataRocks({
container: "#pivotContainer",
toolbar: true,
report: {
dataSource: {
filename: "https://cdn.webdatarocks.com/data/data.csv",
},
slice: {
rows: [
{
uniqueName: "Country",
},
],
columns: [
{
uniqueName: "Measures",
},
],
measures: [
{
uniqueName: "Price",
aggregation: "sum",
},
],
},
},
reportcomplete: function() {
pivot.off("reportcomplete");
createChart();
},
});
let chart;
function createChart() {
pivot.getData(
{},
drawChart,
updateChart
);
};
function drawChart(rawData) {
let chartData = prepareData(rawData);
const config = {
type: "polarArea",
data: {
labels: chartData.labels,
datasets: [{
data: chartData.values,
}],
},
}
chart = new Chart(
document.getElementById("chartContainer"),
config,
);
}
function updateChart(rawData) {
chart.destroy();
drawChart(rawData);
};
function prepareData(rawData) {
let labels = rawData.data.filter(rec => rec.r0 && rec.v0).map(rec => rec.r0);
let values = rawData.data.filter(rec => rec.r0 && rec.v0).map(rec => rec.v0);
return {
labels: labels,
values: values
};
};
</script>
</body>
</html>
Live example
Interact with the following sample where WebDataRocks Pivot Table is integrated with Google Charts to see how they work together:
See also
This step-by-step tutorial will help you integrate WebDataRocks with amCharts. Our tutorial is based on the V4 of amCharts.
Supported chart types
WebDataRocks Connector for amCharts takes the data from the pivot table and prepares it according to the structure of an array of objects required by amCharts. As a result, any chart type will work with WebDataRocks Pivot Table.
Here is the list of demos that show how to integrate different chart types with WebDataRocks:
- Column Chart (demo)
- Line Chart (demo)
- Stacked Column Chart (demo)
- Bar Chart (demo)
- Clustered Bar Chart (demo)
- Stacked Bar Chart (demo)
- Radar Chart (demo)
- Bubble Chart (demo)
- Pie Chart (demo)
- Semi-circle Pie Chart (demo)
- Donut Chart (demo)
- Nested Donut Chart (demo)
- Radar Chart with switched axes (demo)
Follow the steps below to start creating interactive data visualizations.
Step 1. Add WebDataRocks to your project
Step 1.1. Complete the integration guide. Your code of the component with WebDatarocks should look similar to the following:
import { Component } from "@angular/core"; import { WebdatarocksPivotModule } from "@webdatarocks/ngx-webdatarocks"; @Component({ selector: "app-root", standalone: true, imports: [WebdatarocksPivotModule], templateUrl: "./app.component.html", styleUrls: ["./app.component.css"] }) export class AppComponent { }
<app-wbr-pivot [toolbar]="true"> </app-wbr-pivot>
Step 1.2. Create a report for WebDataRocks — connect to the data source and define which fields should be displayed in rows, columns, and measures:
import { Component } from "@angular/core"; import { WebdatarocksPivotModule } from "@webdatarocks/ngx-webdatarocks"; @Component({ selector: "app-root", standalone: true, imports: [WebdatarocksPivotModule], templateUrl: "./app.component.html", styleUrls: ["./app.component.css"] }) export class AppComponent { report = { dataSource: { filename: "https://cdn.webdatarocks.com/data/data.csv", }, slice: { rows: [ { uniqueName: "Country", }, ], columns: [ { uniqueName: "Measures", }, ], measures: [ { uniqueName: "Price", aggregation: "sum", }, ], }, }; }
<app-wbr-pivot [toolbar]="true" [report]="report"> </app-wbr-pivot>
The fields you’ve specified in the report will be shown on the chart.
Step 2. Get a reference to the WebDataRocks instance
Some of WebDataRocks methods and events are needed to create a chart. Using a reference to the WebDataRocks instance, we can access WebDataRocks API.
Import WebdatarocksComponent
and get a reference to the <app-wbr-pivot>
instance using a template variable and the @ViewChild decorator:
import { Component, ViewChild } from "@angular/core"; import { WebdatarocksPivotModule, WebdatarocksComponent } from "@webdatarocks/ngx-webdatarocks"; @Component({ selector: "app-root", standalone: true, imports: [WebdatarocksPivotModule], templateUrl: "./app.component.html", styleUrls: ["./app.component.css"] }) export class AppComponent { @ViewChild("pivot") pivotRef!: WebdatarocksComponent; // ... }
<app-wbr-pivot #pivot [toolbar]="true" [report]="report"> </app-wbr-pivot>
Now it’s possible to interact with the component through this.pivotRef.webdatarocks
.
Note. If you are using the legacy @webdatarocks/ng-webdatarocks wrapper, import WebdatarocksComponent
from it.
Step 3. Add amCharts
Step 3.1. Install the amCharts 4 npm package with the following command:
npm install @amcharts/amcharts4
Step 3.2. Import amCharts into your component:
import * as am4core from '@amcharts/amcharts4/core'; import * as am4charts from '@amcharts/amcharts4/charts'; import am4themes_animated from '@amcharts/amcharts4/themes/animated'; // Apply the imported theme am4core.useTheme(am4themes_animated);
Step 3.3. In the HTML template of the component, add a <div>
container for your chart:
<app-wbr-pivot #pivot [toolbar]="true" [report]="report"> </app-wbr-pivot> <div id="amchartsContainer" style="width: 100%; height: 300px"></div>
Step 3.4. Create a variable that will store a chart instance:
chart!: am4charts.XYChart;
Step 3.5. In your package.json
file, add --prod --build-optimizer=false
flags to the "build"
script to disable the build optimizer:
"scripts": {
// ...
"build": "ng build --prod --build-optimizer=false",
// ...
},
Step 4. Show the data from the pivot table on the chart
Step 4.1. Import the WebDataRocks Connector for amCharts:
import "@webdatarocks/webdatarocks/webdatarocks.amcharts.js";
The Connector provides the amcharts.getData() method, which gets data from WebDataRocks and converts it to the format required by amCharts.
Step 4.2. If we call the amcharts.getData() method before WebDataRocks is fully loaded and ready to use, it will return an empty result. To know when WebDataRocks is ready to provide data for the chart, handle the reportcomplete event:
onReportComplete() { // Unsubscribing from reportcomplete // We need it only to track the initialization of WebDataRocks this.pivotRef.webDataRocks.off("reportComplete"); this.createChart(); }
<app-wbr-pivot #pivot [toolbar]="true" [report]="report" (reportcomplete)="onReportComplete()"> </app-wbr-pivot>
Now we know when the data is loaded and the report is ready.
Step 4.3. Implement the createChart()
function. This function will use the amcharts.getData() method from the Connector:
createChart() { this.pivotRef.webDataRocks.amcharts?.getData( {}, // Function called when data for the chart is ready this.drawChart.bind(this), // Function called on report changes (filtering, sorting, etc.) this.drawChart.bind(this) ); }
Step 4.4. Implement the drawChart()
function from the previous step. drawChart()
initializes the chart, sets all the configurations specific for this chart type, and fills it with the data provided by WebDataRocks:
drawChart(chartConfig: any, rawData: any) { // Create chart instance // The selector must match the id of the <div> container for the chart let chart = am4core.create("amchartsContainer", am4charts.XYChart); // Add data processed by Flexmonster to the chart chart.data = chartConfig.data; // Create a category axis for a column chart let categoryAxis = chart.xAxes.push(new am4charts.CategoryAxis()); categoryAxis.dataFields.category = this.pivotRef.webDataRocks.amcharts?.getCategoryName(rawData); // Create value axis chart.yAxes.push(new am4charts.ValueAxis()); // Create and configure series let series = chart.series.push(new am4charts.ColumnSeries()); series.dataFields.categoryX = this.pivotRef.webDataRocks.amcharts?.getCategoryName(rawData); series.dataFields.valueY = this.pivotRef.webDataRocks.amcharts?.getMeasureNameByIndex(rawData, 0); this.chart = chart; }
Learn more about the amcharts.getCategoryName() and amcharts.getMeasureNameByIndex() API calls.
Step 4.5. Set the autoDispose global option to true
. As a result, the chart will be automatically disposed when it is necessary:
am4core.useTheme(am4themes_animated); am4core.options.autoDispose = true;
Step 5. Run the project
Run your project with the following command:
ng serve --open
Open http://localhost:4200/
in the browser to see how the pivot table looks in combination with amCharts.
To see what a real-time interaction is, try experimenting: filter the data, change the measures and the aggregation functions — the results are reflected on the chart at once.
Learn more about available chart configurations in the amCharts Documentation.
Check out the full code
After completing this tutorial, the full code of the integration should look as follows:
import { Component, ViewChild } from '@angular/core'; import { WebdatarocksPivotModule, WebdatarocksComponent } from '@webdatarocks/ngx-webdatarocks'; import * as am4core from '@amcharts/amcharts4/core'; import * as am4charts from '@amcharts/amcharts4/charts'; import am4themes_animated from '@amcharts/amcharts4/themes/animated'; import '@webdatarocks/webdatarocks/webdatarocks.amcharts.js'; am4core.useTheme(am4themes_animated); am4core.options.autoDispose = true; @Component({ selector: 'app-root', standalone: true, imports: [WebdatarocksPivotModule], templateUrl: './app.component.html', styleUrl: './app.component.css' }) export class AppComponent { @ViewChild('pivot') pivotRef!: WebdatarocksComponent; chart!: am4charts.XYChart; report = { dataSource: { filename: "https://cdn.webdatarocks.com/data/data.csv", }, slice: { rows: [ { uniqueName: "Country", }, ], columns: [ { uniqueName: "Measures", }, ], measures: [ { uniqueName: "Price", aggregation: "sum", }, ], }, }; onReportComplete() { this.pivotRef.webDataRocks.off("reportcomplete"); this.createChart(); } createChart() { this.pivotRef.webDataRocks.amcharts?.getData( {}, this.drawChart.bind(this), this.drawChart.bind(this) ); } drawChart(chartConfig: any, rawData: any) { let chart = am4core.create("amchartsContainer", am4charts.XYChart); chart.data = chartConfig.data; let categoryAxis = chart.xAxes.push(new am4charts.CategoryAxis()); categoryAxis.dataFields.category = this.pivotRef.webDataRocks.amcharts?.getCategoryName(rawData); chart.yAxes.push(new am4charts.ValueAxis()); let series = chart.series.push(new am4charts.ColumnSeries()); series.dataFields.categoryX = this.pivotRef.webDataRocks.amcharts?.getCategoryName(rawData); series.dataFields.valueY = this.pivotRef.webDataRocks.amcharts?.getMeasureNameByIndex(rawData, 0); this.chart = chart; } }
<app-wbr-pivot #pivot [toolbar]="true" [report]="report" (reportcomplete)="onReportComplete()"> </app-wbr-pivot> <div id="amchartsContainer" style="width: 100%; height: 300px"></div>
See also
This step-by-step tutorial will help you integrate WebDataRocks with amCharts. Our tutorial is based on the V4 of amCharts.
Supported chart types
WebDataRocks Connector for amCharts takes the data from the pivot table and prepares it according to the structure of an array of objects required by amCharts. As a result, any chart type will work with WebDataRocks Pivot Table.
Here is the list of demos that show how to integrate different chart types with WebDataRocks:
- Column Chart (demo)
- Line Chart (demo)
- Stacked Column Chart (demo)
- Bar Chart (demo)
- Clustered Bar Chart (demo)
- Stacked Bar Chart (demo)
- Radar Chart (demo)
- Bubble Chart (demo)
- Pie Chart (demo)
- Semi-circle Pie Chart (demo)
- Donut Chart (demo)
- Nested Donut Chart (demo)
- Radar Chart with switched axes (demo)
Follow the steps below to start creating interactive data visualizations.
Step 1. Add WebDataRocks to your project
Step 1.1. Complete the integration guide. Your code of the component with WebDatarocks should look similar to the following:
<script setup>
import { Pivot } from "@webdatarocks/vue-webdatarocks";
import "@webdatarocks/webdatarocks/webdatarocks.css";
</script>
<template>
<div>
<Pivot
toolbar
/>
</div>
</template>
Step 1.2. Create a report for WebDataRocks — connect to the data source and define which fields should be displayed in rows, columns, and measures:
<script setup>
import { Pivot } from "@webdatarocks/vue-webdatarocks";
import "@webdatarocks/webdatarocks/webdatarocks.css";
const report = {
dataSource: {
filename: "https://cdn.webdatarocks.com/data/data.csv",
},
slice: {
rows: [
{
uniqueName: "Country",
},
],
columns: [
{
uniqueName: "Measures",
},
],
measures: [
{
uniqueName: "Price",
aggregation: "sum",
},
],
},
};
</script>
<template>
<div>
<Pivot
toolbar
v-bind:report="report"
/>
</div>
</template>
The fields you’ve specified in the report will be shown on the chart.
Step 2. Get a reference to the WebDataRocks instance
Some of WebDataRocks methods and events are needed to create a chart. Using a reference to the WebDataRocks instance, we can access WebDataRocks API.
Get the reference as follows:
<script setup>
// ...
import { ref } from "vue";
const pivotRef = ref(null);
// ...
</script>
<template>
<div>
<Pivot
ref="pivotRef"
toolbar
v-bind:report="report"
/>
</template>
Now it’s possible to interact with the component through pivotRef.value.webdatarocks
.
Step 3. Add amCharts
Step 3.1. Install the amCharts 4 npm package with the following command:
npm install @amcharts/amcharts4
Step 3.2. Import amCharts into your component:
import * as am4core from "@amcharts/amcharts4/core";
import * as am4charts from "@amcharts/amcharts4/charts";
import am4themes_animated from "@amcharts/amcharts4/themes/animated";
// Apply the imported theme
am4core.useTheme(am4themes_animated);
Step 3.3. In the template of the component, add a <div>
container for your chart:
<template>
<div>
<Pivot
ref="pivotRef"
toolbar
v-bind:report="report"
/>
<div id="amchartsContainer" style="width: 100%; height: 300px"></div>
</div>
</template>
Step 3.4. Create a shallowRef variable to store the chart:
import { ref, shallowRef } from "vue";
// ...
const chartRef = shallowRef(null);
Step 4. Show the data from the pivot table on the chart
Step 4.1. Import the WebDataRocks Connector for amCharts:
import "@webdatarocks/webdatarocks/webdatarocks.amcharts.js";
The Connector provides the amcharts.getData() method, which gets data from WebDataRocks and converts it to the format required by amCharts.
Step 4.2. If we call the amcharts.getData() method before WebDataRocks is fully loaded and ready to use, it will return an empty result. To know when WebDataRocks is ready to provide data for the chart, handle the reportcomplete event:
<script setup>
// ...
function reportComplete () {
// Unsubscribing from reportcomplete
// We need it only to track the initialization of WebDataRocks
pivotRef.value.webdatarocks.off("reportcomplete");
createChart();
}
</script>
<template>
<div>
<Pivot
ref="pivotRef"
toolbar
v-bind:report="report"
v-bind:reportcomplete="reportComplete"
/>
<div id="amchartsContainer" style="width: 100%; height: 300px"></div>
</div>
</template>
Now the chart will be created only when the data is loaded and the report is ready.
Step 4.3. Implement the createChart()
function. This function will use the amcharts.getData() method from the Connector:
function createChart() {
pivotRef.value.webdatarocks.amcharts.getData(
{},
// Function called when data for the chart is ready
drawChart,
// Function called on report changes (filtering, sorting, etc.)
drawChart
);
}
Step 4.5. Create a function to draw the chart. drawChart()
initializes the chart, sets all the configurations specific for this chart type, and fills it with the data provided by WebDataRocks:
function drawChart(chartConfig, rawData) {
// Create chart instance
// The selector must match the id of the <div> container for the chart
let chart = am4core.create("amchartsContainer", am4charts.XYChart);
// Add data processed by Flexmonster to the chart
chart.data = chartConfig.data;
// Create category axis for a column chart
let categoryAxis = chart.xAxes.push(new am4charts.CategoryAxis());
categoryAxis.dataFields.category = pivotRef.value.webdatarocks.amcharts.getCategoryName(rawData);
// Create value axis
chart.yAxes.push(new am4charts.ValueAxis());
// Create and configure series
let series = chart.series.push(new am4charts.ColumnSeries());
series.dataFields.categoryX = pivotRef.value.webdatarocks.amcharts.getCategoryName(rawData);
series.dataFields.valueY = pivotRef.value.webdatarocks.amcharts.getMeasureNameByIndex(rawData, 0);
chartRef.value = chart;
}
Learn more about the amcharts.getCategoryName() and amcharts.getMeasureNameByIndex() API calls.
Step 4.6. Set the autoDispose global option to true
. As a result, the chart will be automatically disposed when it is necessary:
am4core.useTheme(am4themes_animated);
am4core.options.autoDispose = true;
Step 5. Run the project
Run your project with the following command:
npm run dev
Open http://localhost:5173/
in the browser to see an interactive dashboard with WebDataRocks and FusionCharts. The column chart shows the data from WebDataRocks and reacts instantly to any changes in the report.
Learn more about available chart configurations in the amCharts Documentation.
Check out the full code
After completing this tutorial, the full code of the component should look as follows:
<script setup>
import { Pivot } from "@webdatarocks/vue-webdatarocks";
import "@webdatarocks/webdatarocks/webdatarocks.css";
import { ref, shallowRef } from "vue";
import * as am4core from "@amcharts/amcharts4/core";
import * as am4charts from "@amcharts/amcharts4/charts";
import am4themes_animated from "@amcharts/amcharts4/themes/animated";
import "@webdatarocks/webdatarocks/webdatarocks.amcharts.js";
am4core.useTheme(am4themes_animated);
am4core.options.autoDispose = true;
const pivotRef = ref(null);
const chartRef = shallowRef(null);
const report = {
dataSource: {
filename: "https://cdn.webdatarocks.com/data/data.csv",
},
slice: {
rows: [
{
uniqueName: "Country",
},
],
columns: [
{
uniqueName: "Measures",
},
],
measures: [
{
uniqueName: "Price",
aggregation: "sum",
},
],
},
};
function reportComplete () {
pivotRef.value.webdatarocks.off("reportcomplete");
createChart();
}
function createChart() {
pivotRef.value.webdatarocks.amcharts.getData(
{},
drawChart,
drawChart
);
}
function drawChart(chartConfig, rawData) {
let chart = am4core.create("amchartsContainer", am4charts.XYChart);
chart.data = chartConfig.data;
let categoryAxis = chart.xAxes.push(new am4charts.CategoryAxis());
categoryAxis.dataFields.category = pivotRef.value.webdatarocks.amcharts.getCategoryName(rawData);
chart.yAxes.push(new am4charts.ValueAxis());
let series = chart.series.push(new am4charts.ColumnSeries());
series.dataFields.categoryX = pivotRef.value.webdatarocks.amcharts.getCategoryName(rawData);
series.dataFields.valueY = pivotRef.value.webdatarocks.amcharts.getMeasureNameByIndex(rawData, 0);
chartRef.value = chart;
}
</script>
<template>
<div>
<Pivot
ref="pivotRef"
toolbar
v-bind:report="report"
v-bind:reportcomplete="reportComplete"
/>
<div id="amchartsContainer" style="width: 100%; height: 300px"></div>
</div>
</template>
See also
This step-by-step tutorial will help you integrate WebDataRocks with amCharts. Our tutorial is based on the V4 of amCharts.
Supported chart types
WebDataRocks Connector for amCharts takes the data from the pivot table and prepares it according to the structure of an array of objects required by amCharts. As a result, any chart type will work with WebDataRocks Pivot Table.
Here is the list of demos that show how to integrate different chart types with WebDataRocks:
- Column Chart (demo)
- Line Chart (demo)
- Stacked Column Chart (demo)
- Bar Chart (demo)
- Clustered Bar Chart (demo)
- Stacked Bar Chart (demo)
- Radar Chart (demo)
- Bubble Chart (demo)
- Pie Chart (demo)
- Semi-circle Pie Chart (demo)
- Donut Chart (demo)
- Nested Donut Chart (demo)
- Radar Chart with switched axes (demo)
Follow the steps below to start creating interactive data visualizations.
Step 1. Add WebDataRocks to your project
Step 1.1. Complete the integration guide. Your code of the component with WebDatarocks should look similar to the following:
import * as WebDataRocksReact from "@webdatarocks/react-webdatarocks";
function App() {
return (
<div>
<WebDataRocksReact.Pivot
toolbar={true}
/>
</div>
);
}
export default App;
Step 1.2. Create a report for WebDataRocks — connect to the data source and define which fields should be displayed in rows, columns, and measures:
function App() {
const report = {
dataSource: {
filename: "https://cdn.webdatarocks.com/data/data.csv",
},
slice: {
rows: [
{
uniqueName: "Country",
},
],
columns: [
{
uniqueName: "Measures",
},
],
measures: [
{
uniqueName: "Price",
aggregation: "sum",
},
],
},
};
return (
<div>
<WebDataRocksReact.Pivot
toolbar={true}
report={report}
/>
</div>
);
}
The fields you’ve specified in the report will be shown on the chart.
Step 2. Get a reference to the WebDataRocks instance
Some of WebDataRocks methods and events are needed to create a chart. Using a reference to the WebDataRocks instance, we can access WebDataRocks API.
Get the reference with the useRef hook:
React + ES6
// ...
import { useRef } from "react";
function App() {
const pivotRef = useRef(null);
// ...
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
/>
</div>
);
}
export default App;
React + TypeScript
// ...
import { RefObject, useRef} from "react";
function App() {
const pivotRef: RefObject<WebDataRocksReact.Pivot | null> = useRef<WebDataRocksReact.Pivot>(null);
// ...
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
/>
</div>
);
}
export default App;
Now it’s possible to interact with the component through pivotRef.current.webdatarocks
.
Step 3. Add amCharts
Step 3.1. Install the amCharts 4 npm package with the following command:
npm install @amcharts/amcharts4
Step 3.2. Import amCharts 4 into your component:
import * as am4core from "@amcharts/amcharts4/core";
import * as am4charts from "@amcharts/amcharts4/charts";
import am4themes_animated from "@amcharts/amcharts4/themes/animated";
// Apply the imported theme
am4core.useTheme(am4themes_animated);
Step 3.3. Where your React element is returned, add a <div>
container for your chart:
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
/>
<div id="amchartsContainer" style={{ width: "100%", height: "300px" }}></div>
</div>
);
Step 3.4. Create a variable for a chart instance using useRef:
React + ES6
import { useRef } from "react";
// ...
function App() {
const pivotRef = useRef(null);
const chartRef = useRef(null);
// ...
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
/>
<div id="amchartsContainer" style={{ width: "100%", height: "300px" }}></div>
</div>
);
}
export default App;
React + TypeScript
import { RefObject, useRef } from "react";
// ...
function App() {
const pivotRef: RefObject<WebDataRocksReact.Pivot> = useRef<WebDataRocksReact.Pivot>(null);
const chartRef = useRef<am4charts.XYChart | null>(null);
// ...
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
/>
<div id="amchartsContainer" style={{ width: "100%", height: "300px" }}></div>
</div>
);
}
export default App;
Step 4. Show the data from the pivot table on the chart
Step 4.1. Import the WebDataRocks Connector for amCharts:
import "@webdatarocks/webdatarocks/webdatarocks.amcharts.js";
The Connector provides the amcharts.getData() method, which gets data from WebDataRocks and converts it to the format required by amCharts.
Step 4.2. If we call the amcharts.getData() method before WebDataRocks is fully loaded and ready to use, it will return an empty result. To know when WebDataRocks is ready to provide data for the chart, handle the reportcomplete event:
React + ES6
const onReportComplete = () => {
// Unsubscribing from reportcomplete
// We need it only to track the initialization of WebDataRocks
pivotRef.current.webdatarocks.off("reportComplete");
createChart();
};
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
reportcomplete={onReportComplete}
/>
<div id="amchartsContainer" style={{ width: "100%", height: "300px" }}></div>
</div>
);
React + TypeScript
const onReportComplete = () => {
// Unsubscribing from reportcomplete
// We need it only to track the initialization of WebDataRocks
pivotRef.current?.webdatarocks.off("reportComplete");
createChart();
};
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
reportcomplete={onReportComplete}
/>
<div id="amchartsContainer" style={{ width: "100%", height: "300px" }}></div>
</div>
);
Now the chart will be created only when the data is loaded and the report is ready.
Step 4.3. Implement the createChart()
function. It will use the amcharts.getData() method from the Connector:
React + ES6
const createChart = () => {
pivotRef.current.webdatarocks.amcharts.getData(
{},
// Function called when data for the chart is ready
drawChart,
// Function called on report changes (filtering, sorting, etc.)
drawChart
);
};
React + TypeScript
const createChart = () => {
pivotRef.current?.webdatarocks.amcharts.getData(
{},
// Function called when data for the chart is ready
drawChart,
// Function called on report changes (filtering, sorting, etc.)
drawChart
);
};
Step 4.4. Implement the drawChart()
function specified in the previous step. drawChart()
initializes the chart, sets all the configurations specific for this chart type, and fills it with the data provided by WebDataRocks:
React + ES6
const drawChart = (chartConfig, rawData) => {
// Create chart instance
// The selector must match the id of the <div> container for the chart
let chart = am4core.create("amchartsContainer", am4charts.XYChart);
// Add data processed by Flexmonster to the chart
chart.data = chartConfig.data;
// Create a category axis for a column chart
let categoryAxis = chart.xAxes.push(new am4charts.CategoryAxis());
categoryAxis.dataFields.category = pivotRef.current.webdatarocks.amcharts.getCategoryName(rawData);
// Create value axis
chart.yAxes.push(new am4charts.ValueAxis());
// Create and configure series
let series = chart.series.push(new am4charts.ColumnSeries());
series.dataFields.categoryX = pivotRef.current.webdatarocks.amcharts.getCategoryName(rawData);
series.dataFields.valueY = pivotRef.current.webdatarocks.amcharts.getMeasureNameByIndex(rawData, 0);
chartRef.current = chart;
};
React + TypeScript
const drawChart = (chartConfig: any, rawData: any) => {
// Create chart instance
// The selector must match the id of the <div> container for the chart
let chart = am4core.create("amchartsContainer", am4charts.XYChart);
// Add data processed by Flexmonster to the chart
chart.data = chartConfig.data;
// Create and configure series for a pie chart
let categoryAxis = chart.xAxes.push(new am4charts.CategoryAxis());
categoryAxis.dataFields.category = pivotRef.current?.webdatarocks.amcharts.getCategoryName(rawData);
// Create value axis
chart.yAxes.push(new am4charts.ValueAxis());
// Create and configure series
let series = chart.series.push(new am4charts.ColumnSeries());
series.dataFields.categoryX = pivotRef.current?.webdatarocks.amcharts.getCategoryName(rawData);
series.dataFields.valueY = pivotRef.current?.webdatarocks.amcharts.getMeasureNameByIndex(rawData, 0);
chartRef.current = chart;
};
Learn more about the amcharts.getCategoryName() and amcharts.getMeasureNameByIndex() API calls.
Step 4.5. Set the autoDispose global option to true
. As a result, the chart will be automatically disposed when it is necessary:
am4core.useTheme(am4themes_animated);
am4core.options.autoDispose = true;
Step 5. Run the project
Run your project with the following command:
npm run dev
Open http://localhost:5173/
in the browser to see how the pivot table looks in combination with amCharts 4.
To see what a real-time interaction is, try experimenting: filter the data, change the measures and the aggregation functions — the results are reflected on the chart at once.
Learn more about available chart configurations in the amCharts Documentation.
Check out the full code
After completing this tutorial, the full code of the integration should look as follows:
React + ES6
import * as WebDataRocksReact from "@webdatarocks/react-webdatarocks";
import { useRef } from "react";
import * as am4core from "@amcharts/amcharts4/core";
import * as am4charts from "@amcharts/amcharts4/charts";
import am4themes_animated from "@amcharts/amcharts4/themes/animated";
import "@webdatarocks/webdatarocks/webdatarocks.amcharts.js";
am4core.useTheme(am4themes_animated);
am4core.options.autoDispose = true;
function App() {
const pivotRef = useRef(null);
const chartRef = useRef(null);
const report = {
dataSource: {
filename: "https://cdn.webdatarocks.com/data/data.csv",
},
slice: {
rows: [
{
uniqueName: "Country",
},
],
columns: [
{
uniqueName: "Measures",
},
],
measures: [
{
uniqueName: "Price",
aggregation: "sum",
},
],
},
};
const onReportComplete = () => {
pivotRef.current.webdatarocks.off("reportComplete");
createChart();
};
const createChart = () => {
pivotRef.current.webdatarocks.amcharts.getData(
{},
drawChart,
drawChart
);
};
const drawChart = (chartConfig, rawData) => {
let chart = am4core.create("amchartsContainer", am4charts.XYChart);
chart.data = chartConfig.data;
let categoryAxis = chart.xAxes.push(new am4charts.CategoryAxis());
categoryAxis.dataFields.category = pivotRef.current.webdatarocks.amcharts.getCategoryName(rawData);
chart.yAxes.push(new am4charts.ValueAxis());
let series = chart.series.push(new am4charts.ColumnSeries());
series.dataFields.categoryX = pivotRef.current.webdatarocks.amcharts.getCategoryName(rawData);
series.dataFields.valueY = pivotRef.current.webdatarocks.amcharts.getMeasureNameByIndex(rawData, 0);
chartRef.current = chart;
};
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
reportcomplete={onReportComplete}
/>
<div id="amchartsContainer" style={{ width: "100%", height: "300px" }}></div>
</div>
);
}
export default App;
React + TypeScript
import * as WebDataRocksReact from "@webdatarocks/react-webdatarocks";
import { RefObject, useRef } from "react";
import * as am4core from "@amcharts/amcharts4/core";
import * as am4charts from "@amcharts/amcharts4/charts";
import am4themes_animated from "@amcharts/amcharts4/themes/animated";
import "@webdatarocks/webdatarocks/webdatarocks.amcharts.js";
am4core.useTheme(am4themes_animated);
am4core.options.autoDispose = true;
function App() {
const pivotRef: RefObject<WebDataRocksReact.Pivot> = useRef<WebDataRocksReact.Pivot>(null);
const chartRef = useRef<am4charts.XYChart | null>(null);
const report = {
dataSource: {
filename: "https://cdn.webdatarocks.com/data/data.csv",
},
slice: {
rows: [
{
uniqueName: "Country",
},
],
columns: [
{
uniqueName: "Measures",
},
],
measures: [
{
uniqueName: "Price",
aggregation: "sum",
},
],
},
};
const onReportComplete = () => {
pivotRef.current?.webdatarocks.off("reportComplete");
createChart();
};
const createChart = () => {
pivotRef.current?.webdatarocks.amcharts.getData(
{},
drawChart,
drawChart
);
};
const drawChart = (chartConfig: any, rawData: any) => {
let chart = am4core.create("amchartsContainer", am4charts.XYChart);
chart.data = chartConfig.data;
let categoryAxis = chart.xAxes.push(new am4charts.CategoryAxis());
categoryAxis.dataFields.category = pivotRef.current?.webdatarocks.amcharts.getCategoryName(rawData);
chart.yAxes.push(new am4charts.ValueAxis());
let series = chart.series.push(new am4charts.ColumnSeries());
series.dataFields.categoryX = pivotRef.current?.webdatarocks.amcharts.getCategoryName(rawData);
series.dataFields.valueY = pivotRef.current?.webdatarocks.amcharts.getMeasureNameByIndex(rawData, 0);
chartRef.current = chart;
};
return (
<div>
<WebDataRocksReact.Pivot
ref={pivotRef}
toolbar={true}
report={report}
reportcomplete={onReportComplete}
/>
<div id="amchartsContainer" style={{ width: "100%", height: "300px" }}></div>
</div>
);
}
export default App;
See also
If you want to pick up where you left while working with the previous report, you can load it into the pivot table:
Loading the report via the Toolbar
To load a local report
- Go to the Open tab () on the Toolbar.
- Select Local report.
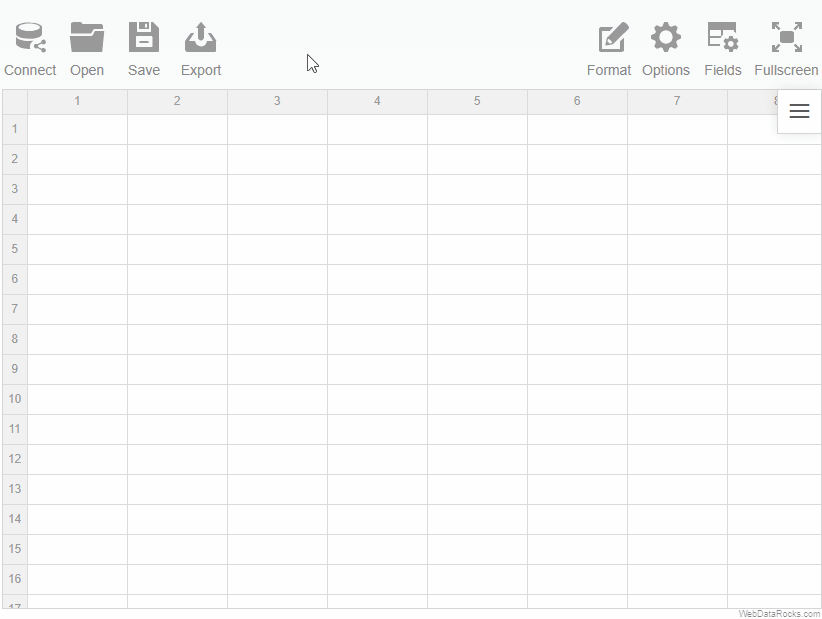
To load a remote report
- Go to the Open tab () on the Toolbar.
- Select Remote report.
- Enter the URL of the remote report.
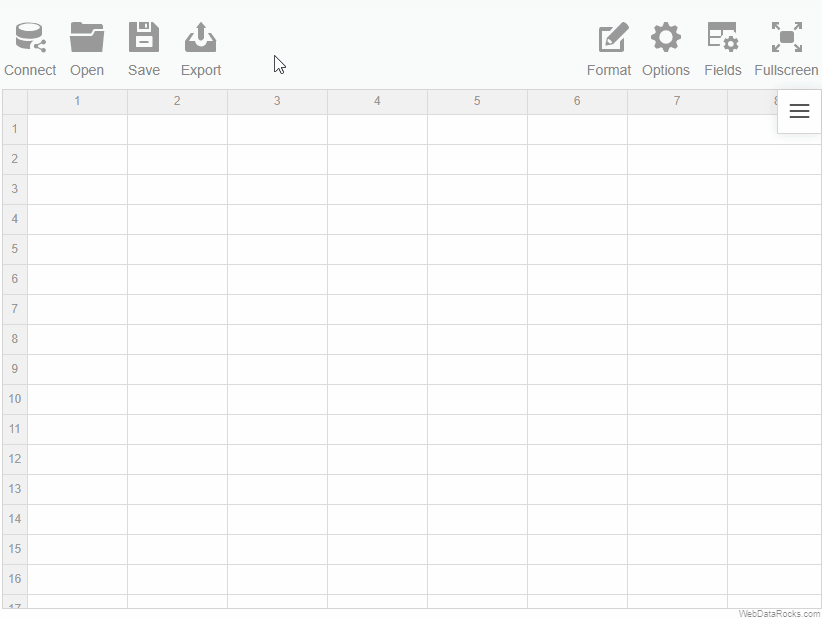
Loading the report programmatically
Load your report while embedding WebDataRocks by specifying a path to your report file:
<script setup>
import { Pivot } from "@webdatarocks/vue-webdatarocks";
import "@webdatarocks/webdatarocks/webdatarocks.css";
</script>
<template>
<div>
<Pivot
report="https://cdn.webdatarocks.com/reports/report.json"
toolbar
/>
</div>
</template>
If you want to pick up where you left while working with the previous report, you can load it into the pivot table:
Loading the report via the Toolbar
To load a local report
- Go to the Open tab () on the Toolbar.
- Select Local report.
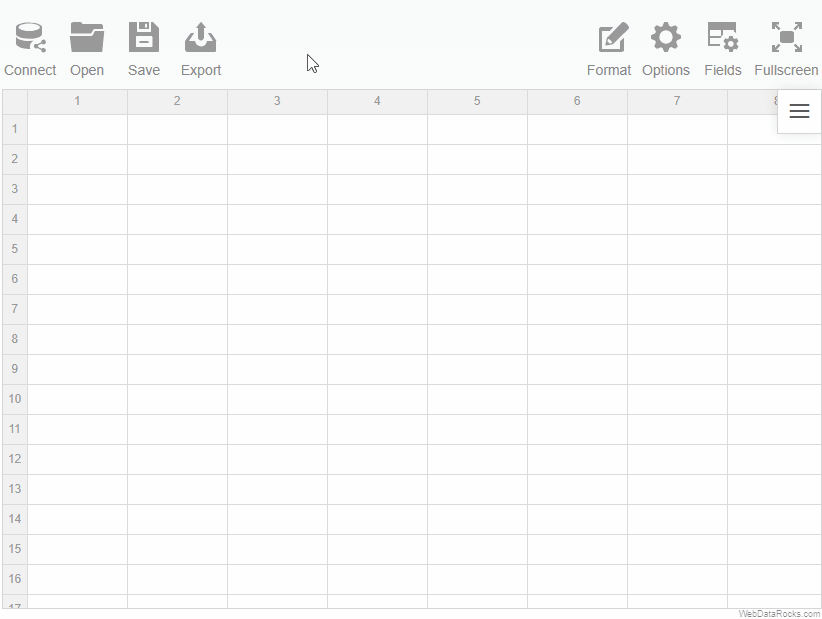
To load a remote report
- Go to the Open tab () on the Toolbar.
- Select Remote report.
- Enter the URL of the remote report.
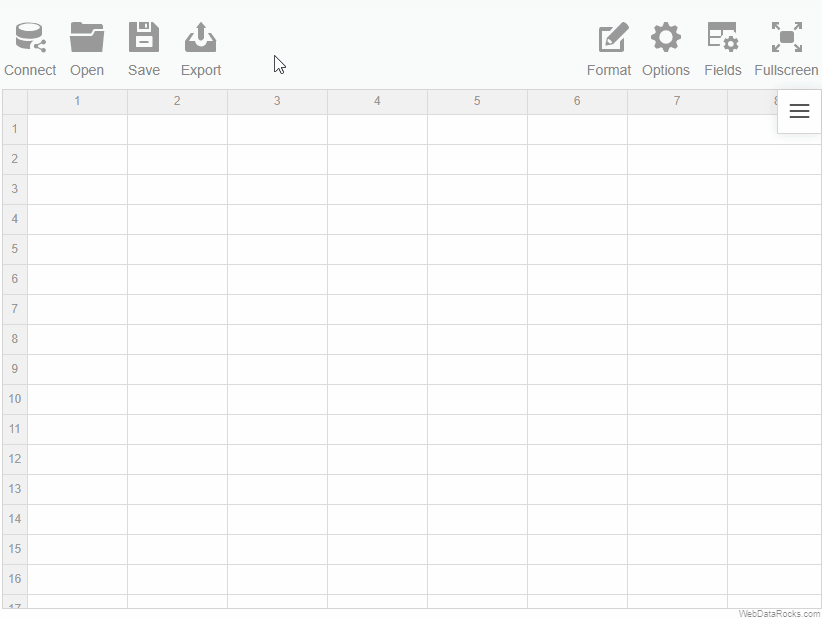
Loading the report programmatically
Load your report while embedding WebDataRocks by specifying a path to your report file:
<app-wbr-pivot
[toolbar]="true"
[report]="https://cdn.webdatarocks.com/reports/report.json">
</app-wbr-pivot>
WebDataRocks Toolbar is designed to make your web reporting experience easier and more convenient. You can customize the Toolbar in the following ways:
- Add new custom tabs.
- Remove the tabs you don’t need.
- Reorder/change the existing tabs.
- Set your custom icons for tabs.
Important Before customizing the Toolbar, ensure it is enabled.
Let’s see how to customize the Toolbar in practice.
All customization can be made using the beforetoolbarcreated event which is triggered before the Toolbar is created. The event’s handler will be responsible for changing the Toolbar.
How to remove tabs
Step 1. Assign a handler (e.g., customizeToolbar
) to the beforetoolbarcreated event. The handler function has the toolbar
as a parameter that contains information about the Toolbar.
Step 2. Inside the handler (e.g., customizeToolbar
), retrieve all the tabs using the toolbar.getTabs()
method. It returns an array of objects that describe tabs’ properties.
Step 3. To remove a tab, delete a corresponding object from the array of tabs.
Your code should look similar to the following example:
<script setup>
import { Pivot } from "@webdatarocks/vue-webdatarocks";
import "@webdatarocks/webdatarocks/webdatarocks.css";
const customizeToolbar = (toolbar) => {
// Get all tabs from the Toolbar
let tabs = toolbar.getTabs();
if (tabs.length > 0) {
// Delete the Connect tab
tabs = tabs.filter(tab => tab.id !== "wdr-tab-connect");
}
toolbar.getTabs = () => tabs;
};
</script>
<template>
<div>
<Pivot
toolbar
:beforetoolbarcreated="customizeToolbar"
/>
</div>
</template>
How to add new tabs
Step 1. Assign a handler (e.g., customizeToolbar
) to the beforetoolbarcreated event. The handler function has the toolbar
as a parameter that contains information about the Toolbar.
Step 2. Inside the handler (e.g., customizeToolbar
), retrieve all the tabs using the toolbar.getTabs()
method. It returns an array of objects that describe tabs’ properties.
Step 3. Add a new tab to the array of tabs.
Your code should look similar to the following example:
<script setup>
import { Pivot } from "@webdatarocks/vue-webdatarocks";
import "@webdatarocks/webdatarocks/webdatarocks.css";
const customizeToolbar = (toolbar) => {
// Get all tabs from the Toolbar
let tabs = toolbar.getTabs();
toolbar.getTabs = () => {
// Add a new tab
tabs.unshift({
id: "fm-tab-newtab",
title: "New Tab",
handler: yourCustomFunction,
icon: toolbar.icons.open,
});
return tabs;
};
};
const yourCustomFunction = () => {
// Your functionality
}
</script>
<template>
<div>
<Pivot
toolbar
:beforetoolbarcreated="customizeToolbar"
/>
</div>
</template>
Even more advanced customization
In this guide, we mentioned how to remove the Toolbar tabs and how to add a new one. For further customization, you can reorder the tabs, set the custom icons for the tabs, or implement new functionality. We recommend investigating the existing code to understand how the Toolbar works:
- Open the source code of the Toolbar –
webdatarocks.toolbar.js
file. - Find a prototype of the
toolbar.getTabs()
method. - Investigate how this method works.
You can also change the appearance of the Toolbar by changing the component theme.
See also
WebDataRocks Toolbar is designed to make your web reporting experience easier and more convenient. You can customize the Toolbar in the following ways:
- Add new custom tabs.
- Remove the tabs you don’t need.
- Reorder/change the existing tabs.
- Set your custom icons for tabs.
Important Before customizing the Toolbar, ensure it is enabled.
Let’s see how to customize the Toolbar in practice.
All customization can be made using the beforetoolbarcreated event which is triggered before the Toolbar is created. The event’s handler will be responsible for changing the Toolbar.
How to remove tabs
Step 1. Assign a handler (e.g., customizeToolbar
) to the beforetoolbarcreated event. The handler function has the toolbar
as a parameter that contains information about the Toolbar.
Step 2. Inside the handler (e.g., customizeToolbar
), retrieve all the tabs using the toolbar.getTabs()
method. It returns an array of objects that describe tabs’ properties.
Step 3. To remove a tab, delete a corresponding object from the array of tabs.
Your code should look similar to the following example:
import { Component } from "@angular/core"; import { WebdatarocksPivotModule } from "@webdatarocks/ngx-webdatarocks"; @Component({ selector: "app-root", standalone: true, imports: [WebdatarocksPivotModule], templateUrl: "./app.component.html", styleUrls: ["./app.component.css"] }) export class AppComponent { customizeToolbar(toolbar: any) { // Get all tabs from the Toolbar let tabs = toolbar.getTabs(); toolbar.getTabs = function() { // Delete the Connect tab return tabs.filter((tab: any) => tab.id !== 'wdr-tab-connect'); } } }
<app-wbr-pivot [toolbar]="true" (beforetoolbarcreated)="customizeToolbar($event)"> </app-wbr-pivot>
How to add new tabs
Step 1. Assign a handler (e.g., customizeToolbar
) to the beforetoolbarcreated event. The handler function has the toolbar
as a parameter that contains information about the Toolbar.
Step 2. Inside the handler (e.g., customizeToolbar
), retrieve all the tabs using the toolbar.getTabs()
method. It returns an array of objects that describe tabs’ properties.
Step 3. Add a new tab to the array of tabs.
Your code should look similar to the following example:
import { Component } from "@angular/core"; import { WebdatarocksPivotModule } from "@webdatarocks/ngx-webdatarocks"; @Component({ selector: "app-root", standalone: true, imports: [WebdatarocksPivotModule], templateUrl: "./app.component.html", styleUrls: ["./app.component.css"] }) export class AppComponent { customizeToolbar(toolbar: any) { // Get all tabs from the Toolbar let tabs = toolbar.getTabs(); toolbar.getTabs = () => { // Add a new tab tabs.unshift({ id: "fm-tab-newtab", title: "New Tab", handler: () => this.yourCustomFunction(), icon: toolbar.icons.open, }); return tabs; }; } yourCustomFunction() { // Your functionality } }
<app-wbr-pivot [toolbar]="true" (beforetoolbarcreated)="customizeToolbar($event)"> </app-wbr-pivot>
Even more advanced customization
In this guide, we mentioned how to remove the Toolbar tabs and how to add a new one. For further customization, you can reorder the tabs, set the custom icons for the tabs, or implement new functionality. We recommend investigating the existing code to understand how the Toolbar works:
- Open the source code of the Toolbar –
webdatarocks.toolbar.js
file. - Find a prototype of the
toolbar.getTabs()
method. - Investigate how this method works.
You can also change the appearance of the Toolbar by changing the component theme.
See also
WebDataRocks Toolbar is designed to make your web reporting experience easier and more convenient. You can customize the Toolbar in the following ways:
- Add new custom tabs.
- Remove the tabs you don’t need.
- Reorder/change the existing tabs.
- Set your custom icons for tabs.
Important Before customizing the Toolbar, ensure it is enabled.
Let’s see how to customize the Toolbar in practice.
All customization can be made using the beforetoolbarcreated event which is triggered before the Toolbar is created. The event’s handler will be responsible for changing the Toolbar.
How to remove tabs
Step 1. Assign a handler (e.g., customizeToolbar
) to the beforetoolbarcreated event. The handler function has the toolbar
as a parameter that contains information about the Toolbar.
Step 2. Inside the handler (e.g., customizeToolbar
), retrieve all the tabs using the toolbar.getTabs()
method. It returns an array of objects that describe tabs’ properties.
Step 3. To remove a tab, delete a corresponding object from the array of tabs.
Your code should look similar to the following example:
import * as WebDataRocksReact from "@webdatarocks/react-webdatarocks";
function App() {
function customizeToolbar(toolbar) {
// Get all tabs from the Toolbar
let tabs = toolbar.getTabs();
toolbar.getTabs = function() {
// Delete the Connect tab
tabs = tabs.filter(tab => tab.id !== "wdr-tab-connect");
return tabs;
}
}
return (
<div>
<WebDataRocksReact.Pivot
toolbar={true}
beforetoolbarcreated={customizeToolbar}
/>
</div>
);
}
export default App
How to add new tabs
Step 1. Assign a handler (e.g., customizeToolbar
) to the beforetoolbarcreated event. The handler function has the toolbar
as a parameter that contains information about the Toolbar.
Step 2. Inside the handler (e.g., customizeToolbar
), retrieve all the tabs using the toolbar.getTabs()
method. It returns an array of objects that describe tabs’ properties.
Step 3. Add a new tab to the array of tabs.
Your code should look similar to the following example:
import * as WebDataRocksReact from "@webdatarocks/react-webdatarocks";
function App() {
const customizeToolbar = (toolbar) => {
// Get all tabs from the Toolbar
let tabs = toolbar.getTabs();
toolbar.getTabs = () => {
// Add a new tab
tabs.unshift({
id: "war-tab-new tab",
title: "New Tab",
handler: yourCustomFunction,
icon: toolbar.icons.open,
});
return tabs;
};
};
const yourCustomFunction = () => {
// Your functionality
}
return (
<div>
<WebDataRocksReact.Pivot
toolbar={true}
beforetoolbarcreated={customizeToolbar}
/>
</div>
);
}
export default App
Even more advanced customization
In this guide, we mentioned how to remove the Toolbar tabs and how to add a new one. For further customization, you can reorder the tabs, set the custom icons for the tabs, or implement new functionality. We recommend investigating the existing code to understand how the Toolbar works:
- Open the source code of the Toolbar –
webdatarocks.toolbar.js
file. - Find a prototype of the
toolbar.getTabs()
method. - Investigate how this method works.
You can also change the appearance of the Toolbar by changing the component theme.